StringBuilder in C#
StringBuilder is a class in C# that provides a way to efficiently construct or modify strings at runtime. It allows for efficient concatenation, insertion, and deletion of characters in a string without creating new objects, which can be faster and more memory-efficient than working with immutable string objects. The StringBuilder class is defined in the System.Text namespace and offers a variety of methods to perform different string operations.
Here are some of the most commonly used methods of StringBuilder class in C# with examples:
Append Method
StringBuilder.AppendAppend()
This method is used to append characters, strings, numbers, or objects to the end of the current StringBuilder object. It returns the same instance of StringBuilder.
Insert Method
Insert(int index, string value)
This method is used to insert characters, strings, numbers, or objects at the specified position in the StringBuilder object. It returns the same instance of StringBuilder.
Remove Method
StringBuilder.Remove(int start, int length)
This method is used to remove a specified number of characters from the StringBuilder object, starting at the specified position. It returns the same instance of StringBuilder.
Replace Method
StringBuilder.Replace(old_val, new_val)
This method is used to replace all occurrences of a specified character or string with another character or string in the StringBuilder object. It returns the same instance of StringBuilder.
ToString Method | StringBuilder.ToString()
This method is used to convert the StringBuilder object to a string. It returns a string that represents the current contents of the StringBuilder object.
Clear Method | StringBuilder.Clear()
This method is used to remove all characters from the StringBuilder object. It returns the same instance of StringBuilder.
Capacity Property: | StringBuilder.Capacity
This property is used to get or set the maximum number of characters that can be stored in the current StringBuilder object without resizing.
Append Formated String
StringBuilder.AppendFormat()
To append a formatted string to a StringBuilder object in C#, you can use the AppendFormat method.
In the code above, the AppendFormat method is used to format a string with placeholders {0} and {1} for the name and age variables, respectively. The formatted string is then appended to the StringBuilder object sb using the AppendFormat method. Finally, the result is converted to a string using the ToString method and printed to the console.
Note that you can also use the Append method to append a formatted string to a StringBuilder object by first formatting the string using string.Format, and then passing the result to the Append method. However, using AppendFormat can be more efficient and easier to read and maintain.
StringBuilder Performance in C#
StringBuilder can offer significant performance benefits over using string concatenation when dealing with large strings or frequent string manipulations in C#.
When you concatenate strings using the + operator or string.Concat method, a new string object is created in memory for each concatenation operation.
In this example, three string objects are created in memory: "Python, ", "Examples", and "!", and then they are concatenated to create a new string object "Python, Examples!". This can become inefficient and slow when working with large or frequently manipulated strings.
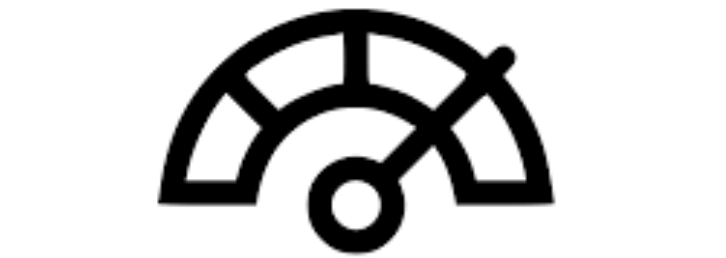
In contrast, StringBuilder uses a resizable buffer that allows for efficient concatenation, insertion, and deletion of characters in a string without creating new objects.
In the above code, a StringBuilder object is used to append each part of the string to the buffer, and then the final result is converted to a string using the ToString method. This is more efficient and faster than concatenating strings using the + operator or string.Concat method.
Difference between StringBuilder and string in C#
In C#, both StringBuilder and string are used for working with strings, but there are some important differences between them.
Mutability | string Vs StringBuilder
The main difference between StringBuilder and string is mutability. A string is an immutable object, meaning its value cannot be changed once it is created. Any operation that appears to modify a string actually creates a new string object, which can cause performance and memory allocation issues if done frequently. In contrast, StringBuilder is a mutable object that allows for efficient manipulation of strings without creating new objects.
Performance | string Vs StringBuilder
As mentioned above, StringBuilder is more performant than string when it comes to frequent manipulations of string values. This is because StringBuilder uses a resizable buffer, which allows for efficient appending, inserting, and deleting of characters, without creating new objects. In contrast, each time a string is modified, a new string object is created in memory, which can lead to performance issues when working with large or frequently modified strings.
Usage | string Vs StringBuilder
string is typically used for storing and manipulating static or constant strings, while StringBuilder is used for constructing or modifying strings that are frequently modified or built dynamically at runtime. For example, string might be used to store a message displayed to the user or to hold the contents of a file that is read once and not modified, while StringBuilder might be used to construct a query or report that is built dynamically based on user input or changing program conditions.
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- How do I create a MessageBox in C#?
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?