Redirect a Web Page to another URL
Redirect a Web Page
Web page redirection, known as URL forwarding or URL redirection, is a fundamental technique in web development that enables a web page to be accessible through multiple URL addresses. For instance, when a user accesses "example.com/page-1" in their browser, they are automatically redirected to "example.com/page-2" instead. This redirection can be within the same website or directed to a different web server or website altogether. The process of redirection is crucial for maintaining user experience, streamlining navigation, and consolidating content across different URLs, thereby improving the website's overall accessibility and usability. There are four main kinds of redirects: 301, 302, HTML Redirect and JavaScript Redirect.
- 301, "Moved Permanently"
- 302, "Found" or "Moved Temporarily"
- HTML Redirect
- JavaScript Redirect
301, "Moved Permanently"
The HTTP response status code 301 Moved Permanently is utilized for permanent URL redirection, signifying that existing links or records using the URL that triggered the response should be updated. The 301 response from the Web server must always include an alternative URL to which redirection should take place. Once received, a Web browser will promptly attempt to access the alternative URL. Employing this method ensures that users and search engines are directed to the appropriate page accurately. The 301 status code indicates that a page has undergone a permanent move to a new location.
302, "Found" or "Moved Temporarily"
The HTTP response status code 302 indicates that the resource being requested has redirected to another resource. When a 302 "Found" or "Moved Temporarily" redirect is encountered, the server redirects the user to the new destination while still utilizing the original location for subsequent requests. Unlike a 301 status code, which denotes a permanent move, a 302 status code signifies a temporary redirection, and the original URL may be used again in the future.
HTML Meta Refresh Redirect
This method uses an HTML meta tag to instruct the browser to automatically redirect to another URL after a specified time interval. It is a simple way to perform a redirection, but it has limitations, such as not being search engine friendly and not allowing much control over the redirection process.
Here's an example of the HTML meta refresh redirect:
In this example, the page will automatically redirect to "https://www.example.com/newpage" after a delay of 5 seconds (specified by content="5").
JavaScript Redirect
JavaScript-based redirection provides more control and flexibility over the redirection process. It allows developers to conditionally redirect users based on certain criteria, such as user agent, cookies, or user behavior. It's commonly used to perform client-side redirection based on specific conditions.
Here's an example of JavaScript-based redirection:
In this example, the JavaScript function redirectToNewPage() is triggered after a delay of 5 seconds using setTimeout(), and it redirects the page to "https://www.example.com/newpage".
Redirect to current URL with URL parameters
The following Javascript code show how to pass parameter while in page redirection
Both window.location and location.href serve different purposes in JavaScript, but they are closely related and often used interchangeably. The window.location object stores comprehensive information about the current document's location, encompassing properties like host, href, port, and protocol. On the other hand, location.href is a string that directly represents the full URL of the current website. It serves as a shorthand for window.location.href. When used as strings, the location object's toString() value and the href property are identical. Consequently, setting window.location is equivalent to setting window.location.href, and both approaches can be used to trigger redirections or change the current page's URL.
The above Javascript code sets the new href (URL) for the current window.
URL Redirection on page loadTo automatically redirect your web page to another webpage upon page load, you can implement the following code. The program displays a brief message for a duration of 3 seconds before initiating the redirection to the specified new page location.
There are situations that if someone wants to redirect back to home page then he can use the following javascript code.
There are some other ways to page redirection in Javascript
This is the same as clicking the "Back button" in your browser, or history.go(-1).
How to redirect your website to its mobile version
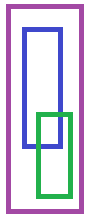
In certain scenarios, there may arise a need to redirect your website to its mobile version. For instance, when a user accesses example.com from their mobile device, it becomes essential to efficiently redirect them to the designated mobile subdomain, such as m.example.com. To facilitate this redirection, you can utilize the following JavaScript code, ensuring a seamless transition to the mobile version of your site.
Note: Normally mobile phones typically have a small screen width, so you should redirect visitors to your mobile site if they have a screen width of less than or equal to 699 pixels.
For iPhones and iPods
The above code only detects iPhone and iPod. The downside of a JavaScript approach is that not all mobile phones support it and people can always turn off JavaScript in their browsers.
Search Engine Optimization and URL redirection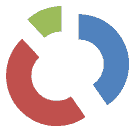
In general, search engines typically utilize the 301 status code to transfer the page rank from the old URL to the new URL during redirection. However, JavaScript redirections return the HTTP response status code 200 OK, which is not considered search engine friendly. As a result, it is advisable to employ alternative redirection methods that yield the status code 301 Moved Permanently. For instance, to inform search engines (SEO) about URL forwarding, it is recommended to incorporate the rel="canonical" meta tag within the website's head section. This is because search engines do not analyze JavaScript to verify redirections, making the use of the rel="canonical" tag a more SEO-friendly approach.
When you use 301 redirect, you show to Search Engine that current page is permanently moved to another location.
The rel="canonical" meta tag is used to address the issue of duplicate content on the web. When a website has multiple pages that share similar or identical content, search engines may interpret it as duplicate content, which can lead to potential penalties in search rankings. By adding the rel="canonical" tag to a page's HTML head section, you indicate to search engines that this particular page is a duplicate or alternative version of a specified "master page." This helps search engines understand the preferred or authoritative version of the content, ensuring that the correct page is indexed and displayed in search results, while avoiding any negative impact on the website's SEO.
- http://example.com/demo-1.html
- http://example.com/category-1/demo-1.html
- http://example.com/category-1/subcategory-1/demo-1.html
The above 3 url are same and exact content. Normaly Search Engines doesn't like the duplicate content and give penalties too. So in this case you should put in every single page a rel canonical tag to the "master" page. For example we will chose "http://example.com/category-1/subcategory-1/demo-1.html".
- How to Print Screen
- How to View Hidden Files in Windows 11, 10, 8 and 7
- Fahrenheit to Celsius Temperatire Conversion Formula
- How to zip files - Compress and uncompress files
- How to Use Robocopy
- How to WMIC ?
- How to recover deleted files
- Microsoft Outlook POP3 Settings, Microsoft Outlook IMAP Settings
- How to Update Windows 11
- What is Three-Tier Architecture ?
- What Is an API (Application Program Interface) ?
- Differences Between HTML4 And HTML5
- How to choose the best antivirus software
- How to Embed a YouTube Video in Your Website
- what is the difference between x64 and x86
- Learn Multiplication of Tables
- What is a Proxy Server?
- How to use a Google Android phone as a Wi-Fi hotspot
- How to Download YouTube Videos
- What is a Phishing Attack ? How can I avoid them?
- What is a Call To Action?
- What's the Difference Between JPG and PNG?
- What Is a "500 Internal Server Error" and How Do I Fix It?
- What is the difference between OTF and TTF fonts
- How to enable flash player on chrome
- How to Select a Video Editing Software
- Why am I getting a "Your connection is not private error" in Chrome
- How to block "Deceptive site ahead" security error?
- Crypto for beginners: What is cryptocurrency?
- What is Bitcoin and how does it work?
- How to fix HTTP Error 502 Bad gateway
- GET url returns "data:text/html,chromewebdata"
- Chrome:Your Clock Is Ahead / Your Clock Is Behind Error
- How to fix ERR_UNKNOWN_URL_SCHEME
- SSL Error on Port 443
- How to Fix This Site Can't Be Reached Error in Chrome
- A disk read error occurred, Press Ctrl+Alt+Del to restart
- How to use System Restore on Windows 10
- What is HTTP error 503 and how do you fix it?
- How to get help in Windows 10
- How To Disable Windows 10 Forced Updates
- How to Fix Google Chrome Error - ERR_SSL_PROTOCOL_ERROR
- How to reset windows 10 password
- What is Blockchain?
- How To Fix: ERR_PROXY_CONNECTION_FAILED
- Unable to send mail through smtp.gmail.com
- How to fix DNS_PROBE_FINISHED_NXDOMAIN
- How to use GTMetrix to Speed up Your Website?
- How to fix System Thread Exception Not Handled Error
- How to fix ERR_INTERNET_DISCONNECTED Error
- WiFi Connected But No Internet Access – How to Fix?
- How to fix a HTTP Error 400: Bad Request?
- What is Deprecation
- How to Fix a 403 Forbidden Error
- What is the maximum length of a URL in different browsers?
- SSL Certificate Problem: Unable to get Local Issuer Certificate
- How to Fix the ERR_CONNECTION_TIMED_OUT Error
- What does localhost:8080 mean?
- How to reduce initial server response time
- 414 Request-URI Too Long - HTTP
- Message channel closed before a response was received