Get domain name from URL
How to Get host domain from URL?
A URI (Uniform Resource Identifier) serves as a standardized representation of a resource that is accessible to your application on the network. It acts as an identifier for locating and retrieving specific resources. Within your application, there are multiple ways to retrieve the host of a URL.
One approach is to utilize the Request object, which provides convenient methods for extracting information about the incoming request, including the host of the URL. Another option is to use the Uri class, which offers a range of properties and methods for parsing and manipulating URIs, including the ability to extract the host component of a given URL. By using these techniques, you can effectively obtain the host information needed for various networking operations within your application.
Get domain name of a url
Using Request.Url
C#Using Uri
C#
VB.Net
Or u can parse the url using regex
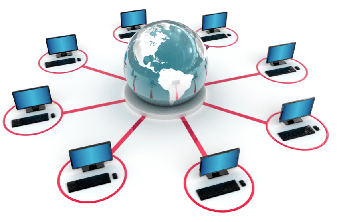
How to parse a domain from URL?
C#
How to get domain part from URL
VB.Net
Extract domain name from URL
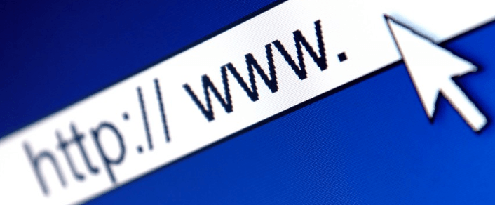
An alternative approach that avoids the need for regular expressions or parsing is to utilize the following code snippet:
VB.Net- Asp.Net Interview Questions (Part-1)
- Asp.Net Interview Questions (Part-2)
- Advantages of ASP.NET Web Development
- What is IIS - Internet Information Server
- What is Virtual Directory
- What is HttpHandler
- Page Directives in Asp.Net
- What is a postback
- What is IsPostBack
- What is global.asax
- Difference between Machine.config and web.config
- Difference between HTML control and Web Server control
- What is Query String
- Difference between Authentication and Authorization
- How to secure Connection Strings
- What is ASP.Net tracing
- Passing values between Asp.Net pages
- Differentiate between client side validation and server side validation
- Adding a Favicon To Your Website
- Asp.Net Textbox value in Javascript
- AutoEventWireup attribute in ASP.NET
- Can I use multiple programming languages in a ASP.net Web Application?
- Difference: Response.Write and Response.Output.Write
- How many web.config files can I have in an application?
- What is Protected Configuration in asp.net?
- Static variablesin .Net , what is their life span?
- Difference between ASP Session and ASP.NET Session?
- What does mean Stateless in Asp.Net?
- What is the Difference between session and caching?
- What are different types of caching using cache object of ASP.NET?
- Which method is used to remove the cache object?
- How many types of Cookies are available in ASP.NET?
- What is Page Life Cycle in ASP.net?
- What is the code behind and Inline Code in Asp.Net?
- What is master page in ASP.NET?
- Can you change a Master Page dynamically at runtime?
- What is cross-page posting in ASP.NET?
- How to redirect a page in asp.net without performing a round trip ?
- How to register custom server control on ASP.NET page?
- How do you validate Input data in Asp.Net?
- What's the difference between ViewData and ViewBag?
- Difference between Response.Redirect and Server.Transfer
- What is the function of the CustomValidator control?
- Define RequiredFieldValidator?
- Difference between custom control and user control
- Difference between Label and Literal control in ASP.Net
- What are the major events in Global.Asax file?
- What is Event Bubbling in asp.net ?
- What is Delay signing?
- What is the difference between in-proc and out-of-proc?
- What is the difference between POST and GET?
- A potentially dangerous Request.Form value was detected from the client