How to handle Java ArithmeticException?
Java ArithmeticException is a runtime exception that occurs when an arithmetic operation encounters an exceptional condition, such as division by zero or an integer overflow. To handle ArithmeticException in Java, you can use try-catch blocks to manage such situations and prevent the program from crashing.
Division by Zero
In this example, the program attempts to divide numerator by denominator, which is zero. This will result in an ArithmeticException. The try-catch block catches the exception and prints an error message. You can include additional error-handling logic or alternative computation within the catch block as needed.
Integer Overflow
In the above example, the program attempts to add 1 to the maximum integer value (maxInt), which will cause an integer overflow, resulting in an ArithmeticException. The try-catch block catches the exception and handles it accordingly.
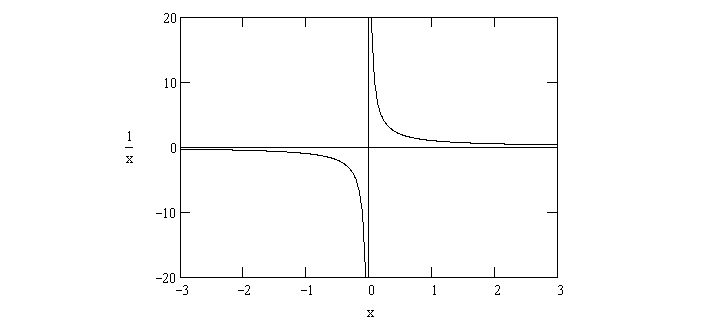
Conclusion
Using try-catch blocks, you can handle Java ArithmeticExceptions and implement custom error-handling logic to recover from exceptional arithmetic situations in your Java programs. This ensures that your applications maintain stability and continue to function correctly even in the face of unexpected arithmetic errors.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException