java.net.SocketException: Connection reset
The SocketException occurs on the server side when the client closes the socket connection abruptly before the server has finished sending the response. This situation is commonly known as a "Connection reset by peer" error.
When a client initiates a socket connection to the server, it establishes a two-way communication channel for sending and receiving data. However, if the client unexpectedly terminates or closes the connection before the server completes sending the response, the server attempts to write data to a socket that is no longer valid. As a result, the server's attempt to write data to the closed socket leads to the SocketException being thrown.
This scenario often occurs when a user quits a browser or interrupts a network request while the server is still processing the response. In such cases, the server receives a signal that the connection has been reset by the client, and the SocketException is raised to indicate this unexpected behavior.
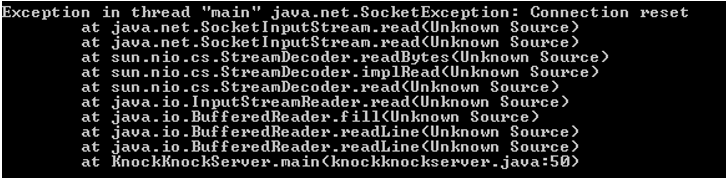
"Connection reset" indicates the receipt of a TCP RST packet, which is a signal from the remote side indicating that the connection for the previous TCP packet is unrecognized, possibly due to closure or an unavailable port. A reset packet contains no payload and has the RST bit set in the TCP header flags, signifying an abrupt termination of the connection. This situation may arise for various reasons and is often associated with the remote side conveying the inability to continue the connection.
Possible causes for the error:
When encountering a java.net.SocketException: Connection reset error in Java, it usually indicates that the remote side (client or server) has closed the TCP connection abruptly. This can happen for various reasons, such as the client or server closing the connection intentionally or due to network issues. To solve this error, you can follow these steps:
- Check the Server-Side Code: If you are running a server application that is responsible for accepting client connections, ensure that your server-side code is correctly handling client disconnections. Make sure you close resources (e.g., input/output streams, sockets) appropriately when a client disconnects.
- Verify Firewall and Network Settings: Sometimes, firewall settings or network issues can lead to connection reset errors. Check your network configuration and ensure that there are no restrictions or firewall rules that may be causing the connection to reset.
- Implement Proper Error Handling: When dealing with network communication, it's essential to implement proper error handling in your code. Catch and handle exceptions appropriately, log any relevant information, and consider implementing retry mechanisms if the connection reset is due to a transient network issue.
- Check Client-Side Code: If you are running a client application that connects to a server, verify your client-side code as well. Ensure that you properly close resources and handle disconnections or errors from the server.
- Investigate Server Logs: If you have access to the server logs, check them for any additional information or error messages that might provide insight into the cause of the connection reset.
- Update Java Version: In some cases, updating to the latest version of Java or using a more stable version may resolve certain networking-related issues.
- Optimize Network Communication: If your application involves frequent network communication, consider optimizing your code to minimize the number of connections and data transfers, which can help reduce the likelihood of encountering connection reset errors.
SocketException
SocketException, as a subclass of IOException, belongs to the category of checked exceptions in Java. As the most encompassing exception within its hierarchy, SocketException serves as a reliable indicator of issues encountered while attempting to open or access a socket. This exception is specifically designed to handle various situations related to socket operations, ensuring that potential errors are caught and addressed appropriately during the execution of the program.The full exception hierarchy of this error is:
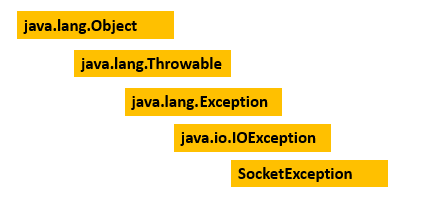
As a best practice in Java programming, it is highly recommended to employ the most precise socket exception class that accurately identifies the encountered problem. Taking advantage of specialized subclasses ensures more targeted and efficient error handling, allowing developers to gain deeper insights into the specific socket-related issues. Additionally, it is essential to acknowledge that SocketException typically accompanies a highly informative error message, offering valuable details about the root cause of the exception. Using this information aids in diagnosing and resolving the underlying situation effectively, contributing to enhanced code quality and overall program robustness.
What is a socket programing?
Socket programming is a fundamental concept in computer programming that utilizes sockets to establish connections and facilitate communication between multiple programs over a network. Sockets act as an interface, enabling applications to interact through the network protocol stack and exchange messages across the network. By using sockets, programmers can create network-enabled applications that can communicate and share data with other programs, both locally and across the internet. Sockets provide the foundation for building various network-based applications, such as web servers, chat applications, and file transfer programs, facilitating seamless data exchange between different entities in the distributed computing environment.
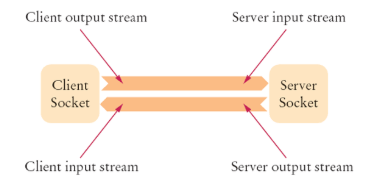
Sockets serve as crucial endpoints in network communications, enabling seamless data exchange between different processes or programs over a network. Typically, a socket server functions as a multi-threaded server, capable of efficiently handling and accepting incoming socket connection requests from clients. In contrast, a socket client refers to a program or process responsible for initiating a socket communication request to establish a connection with the server and facilitate bidirectional data transfer. Understanding the role of sockets in client-server communication is essential for building robust and efficient network applications that use the power of socket-based communication.
Example of a basic Java Socket Programming
Conclusion
Remember that the specific solution may vary depending on the context and details of your application. In some cases, a java.net.SocketException: Connection reset error may be expected behavior due to client disconnections, so handling the exception and recovering from it might be the appropriate action. Always analyze the situation and tailor the solution accordingly.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?