How to handle java.net.SocketTimeoutException
When your Java socket encounters a timeout (throws java.net.SocketTimeoutException: Connection timed out), it indicates that the connection is taking too long to receive a response from the remote device, and as a result, your request expires before a response is received. This situation typically arises when there are delays or issues in the network communication, causing the socket to wait for a response beyond the specified timeout period. To resolve this issue, you can adjust the timeout settings, optimize network performance, or handle the exception in your code to account for potential delays in the communication process.
How to solve?
A developers have the flexibility to pre-set the timeout option for both client and server operations in Java. This capability allows them to control the maximum amount of time a socket connection or communication operation should wait for a response before considering it as timed out. By setting an appropriate timeout value, developers can ensure that their applications do not hang indefinitely when trying to establish connections or receive responses from remote devices.
From Client side:
To efficiently manage timeout scenarios from the client-side, you can define a connection timeout and handle it using a try/catch/finally block. By utilizing the connect(SocketAddress endpoint, int timeout) method, you can set the desired timeout value. This approach allows you to control the waiting time for establishing a connection with the remote endpoint. Within the try block, the connection attempt is made, and if it exceeds the specified timeout, a SocketTimeoutException will be thrown. This exception can be caught in the catch block, enabling you to implement appropriate error handling or fallback strategies. Finally, the finally block can be utilized to perform cleanup actions or any necessary post-connection routines. This systematic approach helps maintain a robust and responsive client-server communication mechanism in Java applications.
Note: If the timeout elapses before the method returns, it will throw a SocketTimeoutException.
If you are using OkHttp Client then you can add:
If you are using OkHttp3 then you must do it using the builder:
Using try/catch/finally
As a developer, you can effectively manage socket connections in your code by encapsulating the relevant code within a try/catch/finally block and handling errors in the catch section. In the try block, you attempt to establish the socket connection. If any exceptions, such as SocketTimeoutException or IOException, occur during the connection attempt, they will be caught in the catch block. In this catch block, you have the opportunity to implement appropriate error-handling strategies.
Depending on the nature of the error and the requirements of your application, you can take various actions in response to the connection failure. For example, you might choose to try connecting a second time to the same socket or attempt to connect to an alternative socket as a backup option. Alternatively, you may decide to handle the error by exiting the program and informing the user about the connection issue. This can help ensure a smoother user experience and avoid unexpected program crashes.
Additionally, the finally block can be used to execute cleanup code or release any resources associated with the socket connection, irrespective of whether the connection attempt was successful or not. This practice helps maintain proper resource management and avoids potential memory leaks.
From Server side:
From the server-side, you can utilize the setSoTimeout(int timeout) method to specify a timeout value for the ServerSocket. This timeout value determines the maximum duration that the ServerSocket.accept() method will block while waiting for a client to establish a connection. By setting an appropriate timeout, you can control how long the server will wait for incoming connection requests before moving on to other tasks or handling potential timeout scenarios.
How to Java SocketTimeoutException?
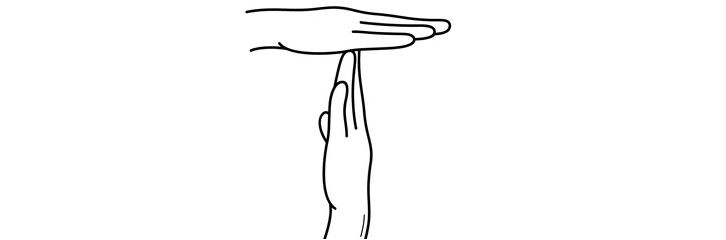
A socket serves as an endpoint for communication between two computer applications. To establish a connection between a remote client and the server, the socket constructor is called, resulting in the instantiation of a socket object. This process temporarily blocks other operations until a successful connection is established. Nevertheless, if the connection attempt fails within a specified time frame, the program raises a ConnectionException with an accompanying error message. This mechanism allows developers to handle connection issues and implement appropriate error-handling strategies in their Java applications.
This exception is occurring on following condition.
- Server is slow and default timeout is less, so just put timeout value according to you.
- Server is working fine but timeout value is for less time. so change the timeout value.
It is crucial to understand that even after this exception is thrown, the socket remains valid, allowing you to retry the blocking call or perform any necessary actions with the valid socket object. To prevent encountering this exception, an alternative approach is to keep the connection alive using the Socket.setKeepAlive() method. By employing this method, you can maintain a persistent connection between the client and the server, thereby mitigating the chances of timeouts or connection issues. Additionally, it is worth noting that the absence of using the setTimeout() method implies an unlimited blocking behavior for receiving data, which can lead to potential issues if not managed effectively.
Conclusion
Setting appropriate timeout values and utilizing the setKeepAlive() method wisely, developers can establish robust and reliable socket connections in their Java applications, ensuring optimal communication between client and server components.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to handle the ArithmeticException in Java?