Java Error: illegal start of expression
Expressions play a fundamental role as building blocks in Java programs, serving as crucial components for computation and logic. One of the frequent and significant compile-time errors encountered in Java is the "illegal start of expression," which arises when the compiler encounters an improper statement within the source code. This error can manifest in various scenarios, signaling the need for careful attention and validation during the coding process.In this course, we'll see examples that elaborate the main causes of this error and how to fix it.
- Missing curly braces
- Method Inside of Another Method
- Access Modifier Inside Method
- Char or String Without Quotes
Missing curly braces
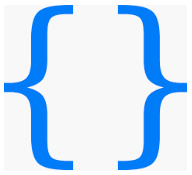
In Java programming, curly braces serve as delimiters to encapsulate meaningful units of code. Each opening curly brace signifies the beginning of a new scope, often nested within other scopes. A crucial aspect to grasp is that variables defined within a particular scope, delimited by opening and closing curly braces, will not be accessible outside of that scope. Thus, the absence of necessary curly braces may result in the "illegal start of expression" error, signifying that the compiler encountered an incomplete or improperly structured expression. Properly enclosing code blocks with curly braces is vital to ensure correct scoping and avoid syntax errors.
exampleMissing the closing curly brace of main() method is the root cause of the problem.
In order to fix this problem, adding the closing curly brace to the main() method.
Method Inside of Another Method (Nested Methods)
In Java, directly nested methods are not supported. Unlike some functional languages and certain JVM languages like Scala and Clojure, Java does not allow methods to be directly defined within other methods. This restriction is due to the fact that methods in Java do not have their own scope; they are contained within a class.
Java's approach to method resolution is based on the inheritance hierarchy of the class that contains the methods. The Java runtime looks up method names within the context of the class or instance that holds them, without applying scoping rules as would be the case for variables. Therefore, attempting to use a method inside another method in Java would result in the "Illegal start of expression" error, as nested methods are not a supported language feature.
Methods should be defined at the class level and not within other methods. This design aligns with Java's object-oriented nature, where methods are part of the class's behavior and can be accessed by instances or the class itself, depending on their visibility (static or instance methods).
exampleIn the above example, we can see that the method innerMethod() is placed inside another method outerMethod().
outputIn java 8 and newer version you can achieve nested methods by lambda expression .
Access Modifier Inside Method
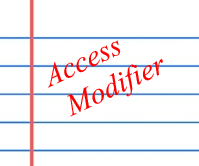
Access modifiers help control the visibility of these elements, ensuring that they are accessible only from specific parts of the code.
Local variables are variables declared within a method, block, or constructor and have a limited scope. They are not accessible from outside their defined context, which makes access specifiers like public, private, and protected unnecessary and invalid for local variables. Using access modifiers inside a method or block would indeed lead to the "Illegal start of expression" error, as it violates the language rules.
Access modifiers are only used at the class and member level to control access to classes, fields, and methods from different parts of the code. Local variables, on the other hand, are confined to the specific method or block in which they are declared and do not require access modifiers.
exampleYou can fix this error by removing the private access modifier from "private int sum".
Char or String Without Quotes
In Java, char is a primitive data type, and String is a class representing a sequence of characters. To define a char value, you use single quotes (') around the character, and to define a String, you use double quotes (").
If you forget to enclose a character or a sequence of characters in the proper quotes, the Java compiler will interpret them as variable names rather than character literals or strings. If the compiler cannot find a valid Java variable with that name, it will report the "illegal start of expression" error.
exampleHere, we forgot to add quotes + within single quotes.

The solution to the problem is simple — wrapping + in single-quotes.
Conclusion
Understanding and following the rules for access modifiers in Java, developers can manage the visibility of their code elements effectively and prevent syntax errors like the "Illegal start of expression" error.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?