Reached end of file while parsing
The "Reached End of File While Parsing" error is a compiler error in programming languages that use curly parenthesis (e.g., Java, Python). This error commonly occurs when there are issues with the proper closing of curly parentheses in the code. It suggests that some curly parentheses are either not ending completely or that there might be extra or mismatched curly parentheses in the code, causing the parser to reach the end of the file prematurely and unable to properly interpret the code. Correcting the curly parenthesis placement and ensuring proper pairing will resolve this error and allow the code to compile successfully.

In programming languages like Java, C++, and others, every opening curly brace '{' must have a corresponding closing curly brace '}'. These braces play a crucial role in defining the scope of code blocks, ensuring proper execution flow and maintaining the structure of the program. Incorrectly placed or omitted curly braces can lead to syntax errors and cause the program to malfunction.
Correct way:
Additionally, while indentation is not a requirement for the program's functionality, it plays a vital role in code readability and maintainability. Properly indenting lines of code helps other programmers, including yourself, understand the program's logic and intent, making it easier to collaborate on and modify the codebase in the future. Writing well-indented code supports good programming practices and contributes to creating maintainable, clean, and easily comprehensible software projects.
How to avoid this error?
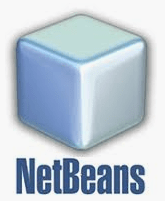
Using a code editor with integrated development environments (IDEs) like NetBeans or Eclipse can significantly aid in avoiding common errors like mismatched braces. These IDEs offer the convenience of autoformatting your code, which can be achieved by pressing Alt+Shift+F or using similar shortcuts. The autoformatting feature ensures that your code is correctly indented and aligns the opening and closing braces with the corresponding control structures such as loops, if statements, methods, or classes. This not only enhances the readability and maintainability of your code but also helps you quickly identify any missing or misplaced braces, preventing syntax errors and streamlining the development process. Using the autoformatting capabilities of IDEs empowers programmers to write cleaner and more error-resistant code, leading to improved productivity and software quality.
Curly Braces in Java
In many programming languages, including Java, C++, JavaScript, and others, the curly brace { is used to indicate the beginning of a block statement. These block statements define a group of one or more statements that are executed together as a single unit. The opening curly brace { is typically followed by a closing curly brace }, which marks the end of the block.
Block statements are essential in programming as they allow you to group related code together and define the scope of variables and functions. They are used extensively in various control structures like if statements, while loops, for loops, do...while loops, switch statements, and more. Properly matching and placing these curly braces is crucial to ensure the correct functioning of the program and to maintain code readability and organization. Mismatched or missing curly braces can lead to syntax errors and unexpected behavior in the program. IDEs and code editors often help in auto-indenting and aligning curly braces, making it easier for programmers to manage block statements and avoid common coding mistakes.
In method or type ( class/interface/enum/annotation ), the { symbol is used to denote the beginning of the body of a class or a method :
It can also be used inside a class to declare an initializer or static initializer block:
In the case of an array literal , the { symbol is used to denote the beginning of the list of elements used inside that literal :
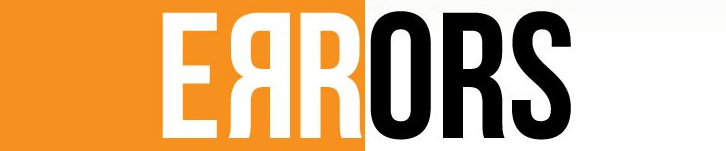
You can find that from above examples, each of these uses of the open brace symbol is different from all the others.
Conclusion
The "Reached End of File While Parsing" error occurs when a compiler or parser encounters the end of a file without finding the expected closing syntax, such as a missing closing curly brace. This error indicates that there is an issue with the structure of the code, often caused by incomplete or mismatched syntax, preventing the successful compilation or parsing of the program.
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?