What is a Java ClassCastException?
The ClassCastException is thrown in Java when your code attempts to perform an invalid type cast, specifically when trying to cast an object to a subclass of which it is not an instance.
In Java, object casting allows you to treat an object as an instance of a different class in the class hierarchy. However, not all types of casting are allowed. If you attempt to cast an object to a subclass of which it is not actually an instance, the ClassCastException is raised at runtime, indicating that the casting operation is not valid.
exampleIn the given example, when trying to cast an Integer to a String, a ClassCastException will be thrown. This is because String is not a subclass of Integer, and the cast is invalid.
The ClassCastException occurs when you attempt to cast an object to a type that it is not compatible with. Casting is only successful when the casted object follows an "is a" relationship to the target type you are trying to cast to. In other words, the object being casted must either be an instance of the target type or one of its subclasses.
Exception hierarchy
All Java errors implement the java.lang.Throwable interface, or are extended from another inherited class therein. The full exception hierarchy of this error is:
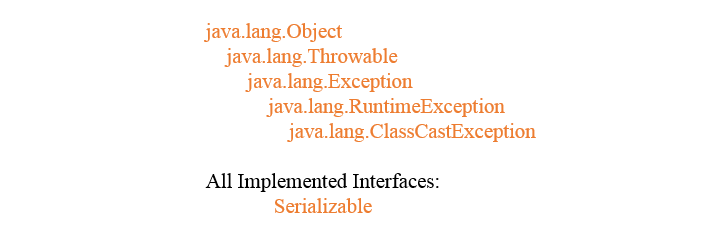
The ClassCastException is a subclass of RuntimeException, which makes it an unchecked exception in Java. Unchecked exceptions do not need to be declared in a method's or constructor's throws clause, providing flexibility to handle or ignore them without forcing the caller to explicitly catch or rethrow the exception.
Being an unchecked exception, the ClassCastException can be thrown during the operation of the JVM without requiring a throws clause in the method signature. This allows developers to handle such exceptions locally within the method or propagate them up the call stack as needed.
When will be ClassCastException is thrown:
- When you try to cast an object of Parent class to its Child class type, this exception will be thrown.
- When you try to cast an object of one class into another class type that has not extended the other class or they don't have any relationship between them.
Casting in Java
Casting refers to the process of converting an object from one type to another. It involves taking an object of a particular class type and treating it as an object of a different class type. The process of converting a variable from one type to another is commonly known as type casting.
Upcasting and Downcasting in Java
Upcasting is casting to a supertype , while downcasting is casting to a subtype . Upcasting is always allowed, but downcasting involves a type check and can throw a ClassCastException.
Here are some basic rules to keep in mind when casting variables:
- Casting an object from a sub class to a super class doesn't require an explicit cast.
- Casting an object from a super class to a sub class requires an explicit cast.
- The compiler will not allow casts to unrelated types.
Even when the code compiles without issue, an exception may be thrown at run time if the object being cast is not actually an instance of that class . This will result in the run time exception ClassCastException .
Downsides of Casting
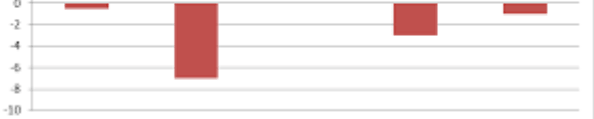
Downcasting carries a certain level of risk because it involves converting an object to a more specialized type, and if done incorrectly, it can result in a ClassCastException at runtime. This is a run-time exception that occurs when the actual object being casted is not compatible with the target type.
When downcasting, it is essential to be well aware of the type of object you are dealing with and perform appropriate checks using the instanceof operator to ensure that the cast is valid. Without proper error handling, a ClassCastException can cause the program to exit or display error messages, affecting the overall user experience.
Conclusion
To avoid such issues, it is crucial to implement error handling mechanisms, such as using try-catch blocks, before downcasting objects. Additionally, following best practices and being cautious when performing downcasting can help prevent unexpected runtime exceptions and ensure the robustness and reliability of your Java program.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?