Int cannot be dereferenced: Java
In Java, there are two main types of variables: primitive types and reference types (objects). Primitive types directly store their values, whereas reference types store references (memory addresses) to objects.
The int type is a primitive type, and it stores the actual value of the integer. It is not an object or a reference to an object. Therefore, there is no need to dereference an int variable, as you can directly access its value.

Dereferencing is the process of accessing the value pointed to by a reference variable (e.g., accessing an object's attributes or invoking its methods using a reference). Since int is not a reference type but a direct value, attempting to dereference it will lead to a compilation error, as it is not applicable to primitive types.
examplePrimitives in Java, including byte, char, short, int, long, float, double, and boolean, are simple value types and do not behave like objects. They do not have member variables or methods, and you cannot perform operations like somePrimitive.something() on them.
In the given example, when x is declared as an int (which is a primitive type), attempting to access a member or method using x.anything is invalid syntax in Java. Since x is a simple value, there is no way to dereference it or access any members or methods associated with it.
Dereferencing is only applicable to reference types (objects), where you can access their attributes and methods using the dot notation. For primitives, you can directly use their values without the need for dereferencing or object-oriented operations.
Differences between primitive types and objects in Java.
Primitive types are designed for efficiency and are not treated as objects. They are stored either as local variables on the stack or as fields (static or non-static) within objects. On the other hand, objects are always allocated on the heap in Java. When you have a local variable that appears to hold an object, it is actually a reference to the object on the heap, not the object itself.
It's crucial to understand that dereferencing is not applicable to primitive types because you can only create references to objects on the heap, not primitive values. Primitive types are not objects and are handled differently from objects in memory allocation and manipulation.
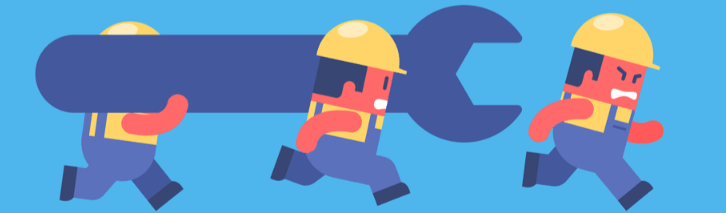
By clarifying these distinctions, your explanation emphasizes the underlying principles of how primitive types and objects are treated and stored in Java, helping to avoid confusion and misunderstandings while writing Java code.
Reference
In computer memory, a reference is an address that points to the location of an object or a variable. When you access the state (attributes/fields) or behavior (methods) of an object using its reference, you are effectively dereferencing the reference to retrieve the actual data or execute the methods stored at that memory location.
The dot (.) operator is used in many programming languages, including Java, to access the members (attributes or methods) of an object through its reference. This process of accessing an object's state or behavior using its reference with the dot operator is indeed known as dereferencing.
Conclusion
Dereferencing involves using the reference to locate the actual data or code stored in memory and is an essential concept in understanding how objects are accessed and manipulated in various programming languages.
- Reached end of file while parsing
- Unreachable statement error in Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?