Unreachable statement error in Java
The "Unreachable Statement" error indicates that certain statements in your program cannot be executed because the control flow does not reach them, despite your assumption that they would be executed. The compiler analyzes the flow of the program and identifies these statements as unreachable, providing error messages to indicate potential logical errors in the code. Addressing these errors is crucial to ensure the correct execution of the program and to avoid unintended behaviors or program crashes.
Java Unreachable statement is an error according to the Java Language Spec .

These statements might be unreachable mostly because of the following reasons:
- Return statement
- Infinite loop
Return statement
In the given example, the return statement will terminate the method, causing any code lines after it to be skipped and remain unexecuted. To ensure the print statement is executed, it should be placed above the return statement within the method. Any statements placed after the return statement become unreachable, as the method has already been exited. The return statement explicitly instructs the program to return control to the calling method and is used to convey a value back to the caller if needed. Careful placement of return statements is essential to maintain the intended control flow and ensure the proper functioning of the program.
Infinite loop
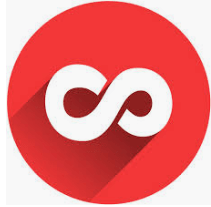
The "Unreachable statement" error occurs because the compiler analyzes the code and identifies certain statements as unreachable. In this case, the statement System.out.print("inside infinite loop"); can never be reached because of the immediate break statement inside the for-loop. The compiler is smart enough to detect such cases of unreachable code, and it raises an error to prevent the generation of bytecode for code that will never be executed. By using a break statement in this scenario, the for-loop is exited prematurely, and any subsequent statements in the loop body are deemed unreachable by the compiler. Properly managing control flow and avoiding unreachable code is crucial to ensure the code's correctness and optimize program performance.
When the compiler reports an unreachable statement, it usually provides information about the specific statement that is unreachable in the code. By following the flow of control from the top of the code to the bottom, developers can analyze and identify why that particular statement can never be reached during program execution.
Conclusion
The rules for determining reachability in Java, as defined in the Java Language Specification 14.21. section 14.21, are designed to be straightforward and easy to evaluate, rather than being 100% accurate. These rules are in place to prevent common programming errors and guide developers in writing valid and logically consistent code. When reasoning about reachability in Java, one must adhere to these defined rules, as common logic may not always apply in certain situations. Abiding by these reachability rules ensures code correctness, improves code readability, and enhances the overall reliability of Java programs.
- Reached end of file while parsing
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?