Class method vs Static method in Python
In Python, both static methods and class methods are used to define methods that are not bound to instances of a class. However, they serve distinct purposes and have different behaviors. Here's a detailed explanation with examples:
Static Methods
Static methods are defined using the @staticmethod decorator. They don't have access to the instance or class itself and work like regular functions that are part of a class's namespace. They can be called using the class name itself without creating an instance.
Class Methods
Class methods are defined using the @classmethod decorator. They receive the class itself as the first argument (usually named cls) and can be used to modify class-level attributes or perform operations that affect the entire class.
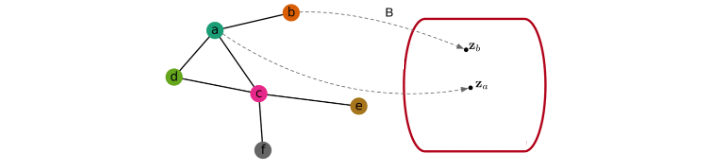
Key Differences
Access to Class Attributes
- Static methods don't have access to instance or class attributes. They work in a similar way to regular functions.
- Class methods can access and modify class attributes through the cls parameter.
Calling Methods
- Static methods are called using the class name itself, like MyClass.static_method().
- Class methods are also called using the class name, like MyClass.class_method().
Use Cases
- Static methods are useful for utility functions that are related to the class but don't require access to instance or class attributes.
- Class methods are used when you need to work with class attributes, create alternate constructors, or perform operations that affect the entire class.
In this example, the class method get_instance_count() accesses the class attribute counter to keep track of the number of instances created. Class methods are especially useful in scenarios where you want to perform actions that impact the entire class and its attributes.
Conclusion
Static methods and class methods in Python are both used to define methods within a class, but they serve distinct purposes. Static methods are independent of instance and class attributes, often used for utility functions, while class methods receive the class itself as a parameter and can modify class attributes or perform operations impacting the entire class. Static methods are called using the class name directly, while class methods also use the class name but have access to class-level attributes through the cls parameter.
- How to use Date and Time in Python
- Python Exception Handling
- How to Generate a Random Number in Python
- How to pause execution of program in Python
- How do I parse XML in Python?
- How to read and write a CSV files with Python
- Threads and Threading in Python
- Python Multithreaded Programming
- Python range() function
- How to Convert a Python String to int
- Python filter() Function
- Difference between range() and xrange() in Python
- How to print without newline in Python?
- How to remove spaces from a string in Python
- How to get the current time in Python
- Slicing in Python
- Create a nested directory without exception | Python
- How to measure time taken between lines of code in python?
- How to concatenate two lists in Python
- How to Find the Mode in a List Using Python