Convert a Python String to int
In the Python programming language, the conversion of a String data type to an integer can be seamlessly achieved through the utilization of the int() method. This method effectively facilitates the transformation process by parsing the provided string representation and carefully crafting an integer equivalent. This conversion procedure assumes a key role in data manipulation scenarios, enhancing the language's versatility in handling diverse data types while maintaining precision and coherence.
int(str)
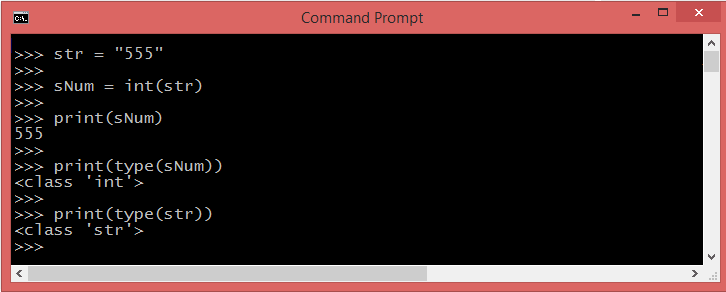
During the course of programming, occasions often arise where the necessity to transform values between distinct data types emerges, enabling the manipulation of data in novel and diverse manners. Python, with its comprehensive features, provides direct mechanisms for type conversions, enabling the seamless transition of data from one data type to another. This functionality proves not only beneficial for everyday programming tasks but also valuable in more demanding and competitive software development endeavors.
Python String to int
A string is defined as a series of one or more characters, while integers represent whole numbers devoid of any fractional element. Within the scope of Python, the process of converting strings into integers can be accomplished using the inherent functionality of the int() method. This method facilitates the seamless transition between these data types, allowing for efficient manipulation and processing.
SyntaxBase specifies the base in which string is if data type is string.
exampleNumber Bases
A number base refers to the assortment of digits or combinations thereof employed by a counting system to denote numerical values. The selection of a specific base involves opting for a whole number exceeding 0. The prevalent and frequently employed numerical system is the decimal system, recognized as base 10, where each digit's positional value is a power of 10.
String to int from different base
The int(str, base) method utilizes the second argument, which signifies the base. Should the string slated for conversion to an integer stem from a number system divergent from base 10, you can explicitly indicate the relevant base for the conversion process. However, it's important to note that the resultant integer, despite originating from a different base, will invariably be represented in base 10.
ast.literal_eval(node_or_string)
To transform a Python string into an integer, you can employ the ast.literal_eval() function. This method is particularly valuable for securely assessing strings containing Python values from potentially untrusted origins, obviating the requirement for manual parsing. However, it's crucial to acknowledge that ast.literal_eval() is not designed to handle highly intricate expressions, such as those encompassing operators or indexing.
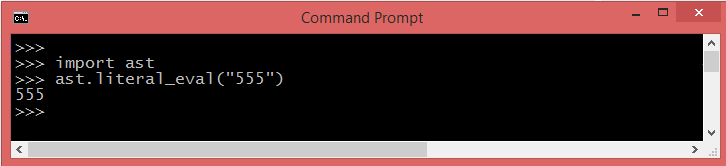
Handling malformed strings
Some strings do not represent valid numbers . If you try to convert such a string, you will get an exception:
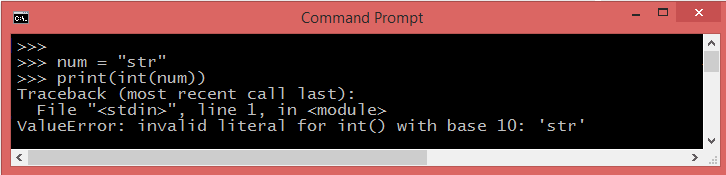
How to fix?
To address the issue of an "invalid literal for int() with base 10" in Python, you can employ the isdigit() method to verify whether a given value constitutes a number. This method yields a Boolean outcome: True if all characters within the string are digits, and False otherwise. By implementing this method, you can ascertain the numeric nature of the input value and avoid errors during integer conversion.
Conclusion
Converting a Python string to an integer involves using the int() function, which facilitates seamless transformation. However, care should be taken when the string contains non-numeric characters, and the isdigit() method can be employed to validate the numeric nature of the string before conversion.
- How to use Date and Time in Python
- Python Exception Handling
- How to Generate a Random Number in Python
- How to pause execution of program in Python
- How do I parse XML in Python?
- How to read and write a CSV files with Python
- Threads and Threading in Python
- Python Multithreaded Programming
- Python range() function
- Python filter() Function
- Difference between range() and xrange() in Python
- How to print without newline in Python?
- How to remove spaces from a string in Python
- How to get the current time in Python
- Slicing in Python
- Create a nested directory without exception | Python
- How to measure time taken between lines of code in python?
- How to concatenate two lists in Python
- How to Find the Mode in a List Using Python
- Difference Between Static and Class Methods in Python?