Python Slice Operator
Python slicing offers an efficient and streamlined approach for systematically retrieving specific segments of data. The use of colons (:) within subscript notation enables the implementation of slice notation, encompassing optional arguments such as start, stop, and step. By utilizing this operator, it becomes feasible to precisely indicate the commencement and conclusion of the slice, along with the incremental step value, facilitating precise control over the extraction of desired portions from sequences.
- start: the beginning index of the slice.
- stop: the ending index of the slice.
- step: the amount by which the index increase.
How slice indexing works?
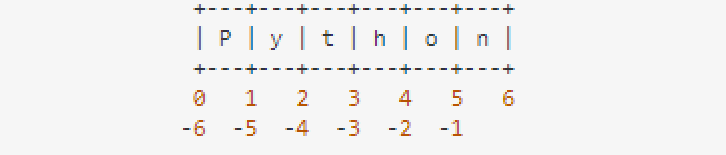
- In forward direction, starts at 0 and ends at len-1.
- In backward direction, starts at -1 and ends at -len.
It is possible to utilize both positive and negative numbers for these parameters. Positive numbers hold a straightforward interpretation, whereas for negative numbers, akin to Python indexes, counting occurs in reverse from the end when referring to start and stop positions. Additionally, when determining the step, the index is decremented.
Basic Slicing
In this example, the slice [7:9] retrieves characters from index 7 up to (but not including) index 9 from the string.
Slicing with Steps
The slice notation start:stop:step allows you to extract elements with a specific step size.
Omitting Indices
Omitting the start index begins the slice from the beginning, and omitting the stop index extends it to the end.
Negative Indices
Negative indices allow you to count from the end. Using [::-1] reverses the sequence.
Conclusion
The slice operator is an essential tool for manipulating sequences in Python, offering a convenient way to access specific portions of data without the need for extensive looping or indexing.
- How to use Date and Time in Python
- Python Exception Handling
- How to Generate a Random Number in Python
- How to pause execution of program in Python
- How do I parse XML in Python?
- How to read and write a CSV files with Python
- Threads and Threading in Python
- Python Multithreaded Programming
- Python range() function
- How to Convert a Python String to int
- Python filter() Function
- Difference between range() and xrange() in Python
- How to print without newline in Python?
- How to remove spaces from a string in Python
- How to get the current time in Python
- Create a nested directory without exception | Python
- How to measure time taken between lines of code in python?
- How to concatenate two lists in Python
- How to Find the Mode in a List Using Python
- Difference Between Static and Class Methods in Python?