How to measure elapsed time in Python?
In Python, multiple modules facilitate the measurement of program execution time. The determination of execution time hinges on factors such as the Operating System, Python version, and the specific interpretation of "time" being considered.
Using time module in Python
The time() function yields the count of seconds elapsed since the epoch. Specifically, the time.time() function from the time module provides this functionality, returning the time in seconds since the epoch. It's important to note that the treatment of leap seconds can vary based on the platform. Utilizing time.time() allows for the measurement of elapsed wall-clock time between two distinct points in a program's execution.
In the above code, the difference between the endTime and startTime, which gives the execution time. It is important to note that, the execution time depends on the Operating System.
Measuring Python execution time in seconds
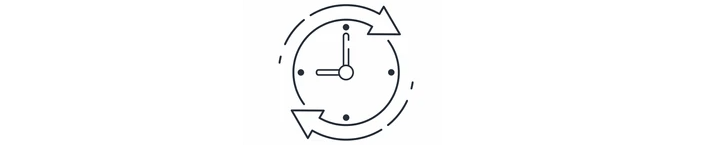
The datetime.timedelta class in Python serves as a means to represent durations, expressing the disparity between two instances of date, time, or datetime down to microsecond precision. This functionality extends to the addition of days, minutes, seconds, hours, weeks, and other units to instances of datetime.date or datetime.datetime. By using datetime.timedelta, you can ascertain execution time in seconds, as illustrated in the provided program. This class proves indispensable for various time-related calculations and measurements.
- On Unix, return the current processor time as a floating point number expressed in seconds. The precision, and in fact the very definition of the meaning of "processor time", depends on that of the 'C' function of the same name.
- On Windows, this function returns wall-clock seconds elapsed since the first call to this function, as a floating point number, based on the Win32 function QueryPerformanceCounter(). The resolution is typically better than one microsecond.
Using timeit module in Python
The Python timeit module furnishes a convenient mechanism to determine the execution time of concise sections of Python code. It adeptly circumvents numerous pitfalls often associated with measuring execution durations. By repeatedly executing your code snippet millions of times (defaulting to 1,000,000), the timeit module yields a statistically significant measurement of code execution time, enabling accurate performance assessment. This module proves invaluable for precise benchmarking and profiling of code segments.
timeit.default_timer
Also you can use timeit.default_timer instead of timeit.timeit. The default_timer provides the best clock available on your platform and version of Python automatically:
The default_timer() function for measurement may encounter interference from concurrent Python programs running on the same system. Hence, when utmost timing accuracy is essential, a recommended practice is to conduct multiple timing iterations and select the optimal result. For Unix systems, time.clock() can be employed to gauge CPU time consumption, providing a distinct perspective on program execution duration. This approach ensures that timing assessments are refined and tailored to specific accuracy requirements, enhancing the reliability of execution time measurements.
Using Datetime Module
The datetime module offers the capability to calculate the time elapsed within a code block, although it's important to note that this approach might not deliver high precision. The resulting output is presented in the format of HH:MM:SS, which represents hours, minutes, and seconds. While this method can provide a general sense of time elapsed, it's advisable to consider alternative methods for more accurate and granular timing measurements when necessary.
Elapsed Time as Days, Hours, Minutes and Seconds
Conclusion
Various methods are available to measure elapsed time during program execution. The time module's time() function can capture seconds since the epoch, while the datetime module's timedelta class offers precise duration calculations. The timeit module facilitates accurate benchmarking, and default_timer() can provide timing insights, although for high precision, repetition and selection of the best result are recommended.
- How to use Date and Time in Python
- Python Exception Handling
- How to Generate a Random Number in Python
- How to pause execution of program in Python
- How do I parse XML in Python?
- How to read and write a CSV files with Python
- Threads and Threading in Python
- Python Multithreaded Programming
- Python range() function
- How to Convert a Python String to int
- Python filter() Function
- Difference between range() and xrange() in Python
- How to print without newline in Python?
- How to remove spaces from a string in Python
- How to get the current time in Python
- Slicing in Python
- Create a nested directory without exception | Python
- How to concatenate two lists in Python
- How to Find the Mode in a List Using Python
- Difference Between Static and Class Methods in Python?