How to remove whitespace from a string in python
In Python, the process of eliminating extraneous whitespace characters from a string involves employing various techniques for whitespace removal. This is essential to enhance the string's cleanliness and uniformity, ensuring a more refined and structured representation. By implementing string manipulation methods and functions specifically designed for whitespace trimming, one can achieve a streamlined and coherent string presentation, thereby enhancing the overall readability and usability of the text-based data. By using the following methods, let's see how to remove whitespaces in a string .
- str.strip()
- str.lstrip()
- str.rstrip()
- str.replace()
- translate()
- re.sub()
Python strip() method
For the purpose of removing leading and trailing spaces within a string, the strip() method serves as the appropriate tool. This function effectively trims the whitespace from both the start and end of the string, resulting in a cleaner and more focused textual representation.
Python lstrip() method
To eliminate spaces exclusively from the start of a string, the lstrip() method is the recommended approach. By employing this method, spaces located at the beginning of the string are effectively eradicated, preserving the content while refining its initial alignment.

Python rstrip() method
Should the objective be the eradication of spaces solely from the end of a string, the optimal course of action involves the utilization of the rstrip() method. By employing this method, any trailing spaces at the conclusion of the string are effectively eliminated, refining the string's presentation while retaining its core content.

All three string functions strip lstrip, and rstrip can take parameters of the string to strip, with the default being all white space.
Python replace() method
For the comprehensive removal of all space characters within a string, the recommended approach is to employ the replace() method. By applying this method, all instances of space characters are systematically substituted, resulting in a string devoid of any spaces. This method is particularly useful when aiming to achieve a whitespace-free representation for further processing or display.

Python translate() method
To effectively eliminate all types of whitespace, encompassing spaces, tabs, and carriage return-line feed (CRLF) characters, an elegant and concise solution involves utilizing the translate() method. This function allows for a one-liner approach to achieving comprehensive whitespace removal, resulting in a refined string representation free from various forms of spacing.
OR if you want to remove only whitespace :
Using Regular Expressions
If you want to remove leading and ending spaces in a string, use strip():
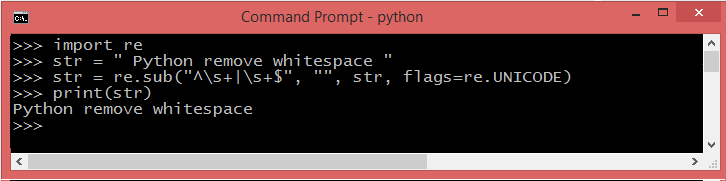
If you want to remove spaces in the beginning of a string , use lstrip():
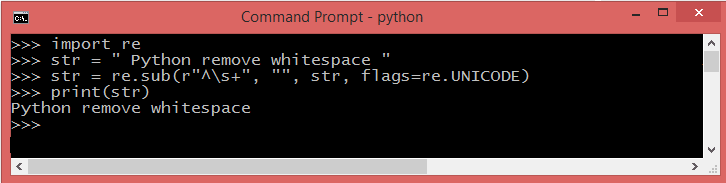
If you want to remove spaces in the end of a string , use rstrip():
If you want to remove all spaces in a string, even between words:
Remove all duplicate whitespaces in the string
To address the removal of duplicated whitespaces and newline characters within a string, a strategic solution involves the combined utilization of the join() function along with the split() function. By employing this approach, one can efficiently eliminate redundant spacing and newline elements, resulting in a more streamlined and coherent string representation. This technique is particularly effective when striving to enhance the legibility and formatting of textual data.
- split(): Returns list of all words in the string separated using delimiter string. If the delimiter is not mentioned, by default whitespace is the delimiter.
- join(): This method takes all items in the iterable and combines them into a string using a separator.
Or
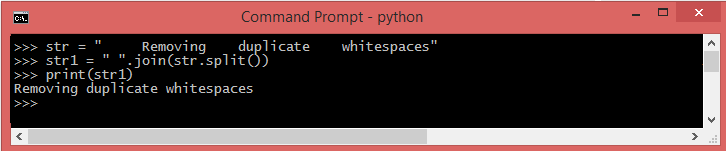
Conclusion
Whitespace removal from a string can be accomplished through various methods. Using strip() eradicates leading and trailing spaces, lstrip() removes spaces from the beginning, and rstrip() eliminates spaces from the end. Alternatively, replace() replaces all space characters, while employing translate() allows for the removal of diverse whitespace forms.
- How to use Date and Time in Python
- Python Exception Handling
- How to Generate a Random Number in Python
- How to pause execution of program in Python
- How do I parse XML in Python?
- How to read and write a CSV files with Python
- Threads and Threading in Python
- Python Multithreaded Programming
- Python range() function
- How to Convert a Python String to int
- Python filter() Function
- Difference between range() and xrange() in Python
- How to print without newline in Python?
- How to get the current time in Python
- Slicing in Python
- Create a nested directory without exception | Python
- How to measure time taken between lines of code in python?
- How to concatenate two lists in Python
- How to Find the Mode in a List Using Python
- Difference Between Static and Class Methods in Python?