C# Message Box
In C#, a MessageBox is a pre-built dialog box that displays a message to the user and typically requires some sort of user interaction, such as clicking a button or entering input. The MessageBox class is part of the System.Windows.Forms namespace, which provides a variety of graphical user interface (GUI) components for building Windows desktop applications.
C# MessageBox
Following is an example of a simple C# MessageBox program that displays a message to the user and waits for the user to click the OK button:
Here's what this program does:
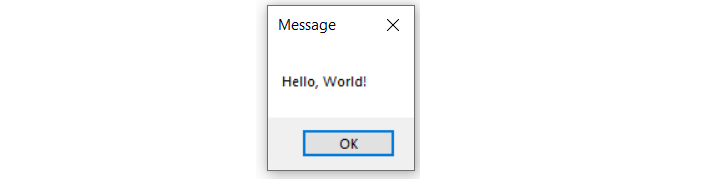
- It imports the System and System.Windows.Forms namespaces.
- It defines a Program class.
- It defines a Main method that is called when the program starts.
- In the Main method, it calls the MessageBox.Show method to display a message box with the message "Hello, World!" and the title "Message".
- It calls the Application.Run method to start a message loop and wait for the user to click the OK button.
When you run this program, a message box will appear with the message "Hello, World!" and the title "Message". The program will wait for the user to click the OK button before exiting.
C# MessageBox with Yes/No buttons
You can create a MessageBox with Yes/No buttons by using the MessageBoxButtons.YesNo argument in the MessageBox.Show() method:
In the above example, the MessageBox.Show() method displays a dialog box with the message "Do you want to continue?" and the title "Confirmation". The MessageBoxButtons.YesNo argument specifies that the dialog box should have Yes/No buttons. The DialogResult variable result stores the user's choice, which can be either DialogResult.Yes or DialogResult.No.
C# MessageBox Custom Button
MessageBox in C# provides an option to create custom buttons with a predefined set of values. To create a custom button, you can use the MessageBoxButtons enumeration. This enumeration provides different types of buttons that can be added to the MessageBox, such as "OK," "Yes," "No," "Cancel," "Retry," and "Ignore."
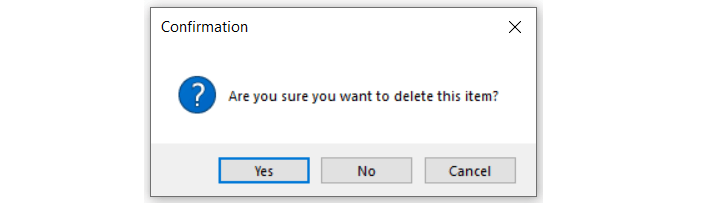
Following is an example of creating a MessageBox with custom buttons:
C# MessageBox with New Line:
You can add a new line to the MessageBox's text by adding "\n" to the string. This will insert a new line in the text.
Following is an example of creating a MessageBox with a new line:
- Advantages of C#
- C# vs. Java: What Are Its Main Differences
- Advantages of C# over Python
- First C# Program | Hello World
- Difference between Console.Write and Console.WriteLine in C#
- C# Comments
- How to reverse a string in C#
- Palindrome in C# with Examples
- Fibonacci Series Program in C# with Examples
- C# Program to Print Number Triangle
- Get Integer Input from User in C# Console Application
- C# StringBuilder | Learn To Use C# StringBuilder Class
- C# HashMap (Dictionary)
- Ternary Operator (? :) in C# with Examples
- How To Sort Datatable in C#
- Struct Vs Class in C# | Differencees and Advantages
- Async And Await In C#
- IEnumerable in C# | Examples
- ref Vs out in C#
- How to remove item from list in C#?
- How to Add Items to a C# List
- C# StreamWriter Examples
- StreamReader in C# |Examples
- C# Map Example
- Static Method In C# | Examples
- How to convert an Enum to a String in C#
- How to convert a string to an enum in C#
- How to filter a list in C#?