What is the cause of NoSuchElementException
In Java, NoSuchElementException is thrown when attempting to access an element from an iterable (such as a collection or iterator) that does not have any more elements to provide. This exception serves as a signal that the requested element does not exist in the current iteration.
The NoSuchElementException typically occurs when using methods like next() in an iterator, which returns the next element in the iteration sequence. If there are no more elements to provide, the next() method throws this exception to indicate that the iteration has reached its end.
exampleHow to solve NoSuchElementException?
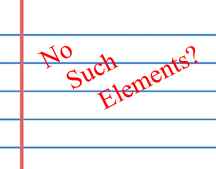
To avoid the NoSuchElementException, it's essential to use the hasNext() method to check if there are more elements in the iteration before calling the next() method. The hasNext() method returns a boolean value indicating whether the iteration has any more elements.
By checking the result of hasNext() before calling next(), you ensure that the iteration has not reached its end, and it's safe to retrieve the next element using next(). If hasNext() returns true, then you can confidently call next() to get the next element. If hasNext() returns false, it means there are no more elements in the iteration, and calling next() would result in a NoSuchElementException. The following methods are used to check the next position:
- hasNext()
- hasMoreElements()
This way ensures that any element is being accessed if it exists.
java.util.NoSuchElementException
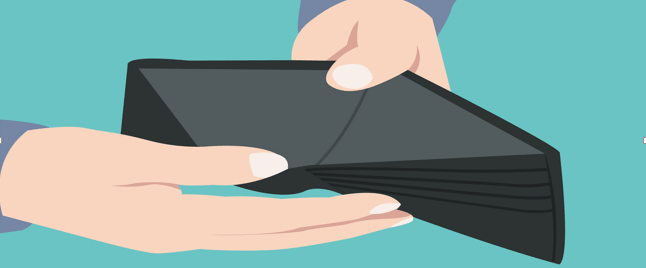
An exception is an abnormal condition that occurs during the execution of a program, disrupting the normal flow of code execution. Exceptions can happen for various reasons, such as invalid input, division by zero, accessing elements beyond the limit, or any other unexpected situation that violates the program's normal behavior.
The NoSuchElementException is indeed an unchecked exception in Java. Being an unchecked exception, it does not need to be declared in a method's or a constructor's throws clause. Unchecked exceptions, including NoSuchElementException, are not required to be explicitly caught or declared in the method signature, providing flexibility in handling exceptions locally within the code.
Unchecked exceptions are generally used for runtime exceptions, indicating issues that can be detected only during program execution. The Java compiler does not enforce explicit handling of unchecked exceptions, making it the responsibility of the developer to handle these exceptions based on the specific requirements of their code. The full exception hierarchy of this error is:
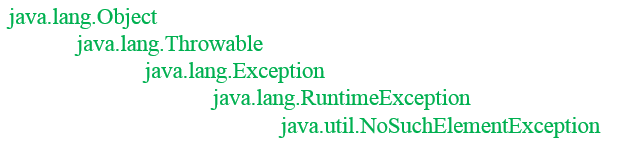
The java.util.NoSuchElementException is a RuntimeException which can be thrown by different classes in Java like Iterator, Enumerator, Scanner or StringTokenizer . It is thrown by the following methods:
- nextElement() of Enumeration interface
- next() of NamingEnumeration interface
- nextElement() of StringTokenizer class
- next() of Iterator interface
Similarly, the previous() method of the ListIterator returns the previous element of the collection, if this method is invoked on an empty object or at the stating position of it a NoSuchElementException is generated at the runtime.
Conclusion
Handling exceptions properly in a program is essential to ensure robustness and smooth error recovery. By catching and handling exceptions appropriately, you can improve the reliability and user experience of your Java applications.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- Error: Could not find or load main class in Java
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?