Error: Could not find or load main class
In Java programming, the presence of a main() method is essential for the execution of programs, as it serves as the entry point where the program's execution commences. However, on occasion, when running a Java program, you may encounter the error "Could not find or load main class."
This error arises when attempting to execute the main() method directly within the class using the java command, which is an incorrect approach. The java command should be followed by the name of the compiled class file containing the main() method, ensuring proper execution and avoiding the aforementioned error. Understanding and adhering to the correct syntax for running Java programs with main() methods is crucial for smooth program execution and error-free operation.
Reasons to Occur Error :
- File Extension
- Wrong package
- Invalid Classpath
- Wrong Class Name
File Extension
Source code files (.java extension) are transformed into compiled .CLASS files using the Java compiler (javac command). These .CLASS files contain bytecode that is executable by the Java Virtual Machine (JVM). For example, if the Java source code file is named "MyClass.java," the resulting compiled code will be stored in "MyClass.class." This compilation process is a crucial step in the execution of Java programs, as it transforms human-readable code into a format that can be interpreted and executed by the JVM, ensuring platform independence and portability.
You will get that error if you call it using:
Instead, use this:
Wrong package

A package is a mechanism used to group related classes together, providing a way to organize and manage code effectively. Packages help avoid naming conflicts, as they allow developers to create classes with the same name in different packages without collision. Additionally, using packages leads to more maintainable code by promoting modularization and encapsulation of functionalities.
When running a Java class that is part of a package, the fully qualified name of the class must be provided to ensure its proper execution. The fully qualified name includes the package name followed by the class name, separated by periods. This way, the Java runtime can accurately locate and execute the desired class within the specified package, contributing to the overall efficiency and structure of the Java program. When the Main class is inside a package then you need to run it as follows :
Then calling:
You will get "Error: Could not find or load main class MainClassName" . This is because it must be called with its fully qualified class name:
Here you can see, the name of this class is not MainClassName, It's packageName.MainClassName . Attempting to execute MainClassName does not work, because no class having that name exists. Not on the current classpath anyway.
Invalid Classpath
The classpath in Java refers to the specific path where the Java Virtual Machine searches for user-defined classes, packages, and resources during program execution. If you encounter an error despite correctly declaring the classname, it is likely that the Java command cannot locate the specified classname in the given path. To resolve this issue, it is essential to ensure that you include the location of your .class file in the classpath. For instance, if the file is in the current folder, you can add the current directory (denoted by a dot, ".") to your classpath. By setting the classpath appropriately, you enable the Java Virtual Machine to find and load the required classes and resources, ensuring smooth execution and error-free operation of your Java programs.
For example, if your class file "MyClass" is saved in the following directory d:\temp
Or
In this example, . (dot) meaning the current directory, is the entirety of classpath.
If you're running it from command prompt , you need to make sure your classpath is correct. If you set it already, you need to restart your terminal to reload your system variables.
Case Sensitive :
In Java, it is crucial to be aware that the language is case sensitive. The compiler will not raise any complaints since the code is technically correct, and it will generate a class file that precisely matches the class name. For instance, if the Java source code file is named "MyClass.java," the resulting compiled code will be stored in "MyClass.class." However, when attempting to run the program using the name "myClass," an error message will be encountered, as there is no corresponding file named "myClass.class." This underscores the significance of accurately specifying the class name, including its case, when running Java programs to ensure proper execution and avoid such errors.
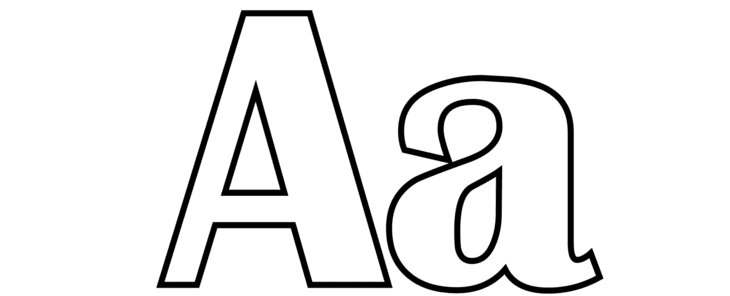
Wrong Class Name
This error can occur when there is a mismatch between the Java file name (.java) and the main class name. Java requires that the filename and the main class name within the file should be the same. If there is a discrepancy in the names, it will lead to the error "Error: Could not find or load main class," as the Java Virtual Machine cannot locate the appropriate main class to execute. Ensuring consistency between the Java file name and the main class name is essential for the successful compilation and execution of Java programs.
exampleIf you save the above program in a "sample.java" file and compile it, the compiler generate "MyClass.class" file and not "sample.class".
When you run
It failed with the error "Could not find or load main class sample ".
So, you have to call
Conclusion
By following the above steps, you can troubleshoot and resolve the "Error: Could not find or load main class" and ensure smooth execution of your Java programs.
- Reached end of file while parsing
- Unreachable statement error in Java
- Int Cannot Be Dereferenced Error - Java
- How to fix java.lang.classcastexception
- How to fix java.util.NoSuchElementException
- How to fix "illegal start of expression" error
- How to resolve java.net.SocketException: Connection reset
- Non-static variable cannot be referenced from a static context
- How to Handle OutOfMemoryError in Java
- How to resolve java.net.SocketTimeoutException
- How to handle the ArithmeticException in Java?