Python Operators
In software programming, an operator is a symbol that signifies an action or process. For instance, the "+" symbol functions as an arithmetic operator, representing addition. These symbols were derived from mathematics and logic and are supported by various programming languages. Operators form the foundation of any program, enabling a wide range of tasks, from basic counting functions to intricate algorithms like security encryption.
In Python, operators are distinct symbols used to signify specific computations or operations. Python includes operators in the following categories:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Boolean Operators
- Bitwise Operators
- Membership Operators
- Identity operators

Python Arithmetic Operators
Arithmetic operators play a crucial role in mathematical expressions, enabling the manipulation of numerical values, whether in the form of literals or variables. When used, these operators diligently process their operands and deliver a singular numerical outcome, serving as the foundation for performing various mathematical computations in programming.
Operator | Description | Syntax |
---|---|---|
+ | Addition | x + y |
- | Subtraction | x - y |
* | Multiplication | x * y |
/ | Division | x / y |
% | Modulus | x % y |
** | Exponentiation | x ** y |
// | Floor division | x // y |
Python Addition (+) Operator
Addition is a fundamental arithmetic operation that involves combining two or more numbers to yield their total sum. In other words, it represents the act of adding quantities together to obtain a unified result.
Python Subtraction (-) Operator
Subtraction is an essential arithmetic operation that entails deducting the value on the right side from the one on the left side. In this process, the two values are compared, and the difference between them is computed.
Python Multiplication (*) Operator
Multiplication is a fundamental arithmetic operation that involves calculating the product of the values on either side of the operator. In this operation, the two values are multiplied together to obtain the final result.
Python Division (/) Operator
Division is a fundamental arithmetic operation used to distribute a group of objects or values into equal parts. It involves partitioning a quantity into separate and equal portions, ensuring a fair and balanced allocation.
Python Modulus (%) Operator
The modulo operator, often denoted as "mod" or "%", is a fundamental arithmetic operation used to calculate the remainder when one number is divided by another. It provides the remaining value after the division process, which can be useful in various mathematical and programming scenarios, such as determining even or odd numbers or implementing cyclic behaviors in algorithms.
Python Floor division (//) Operator
The floor division operator (//) is another arithmetic operation used in Python. It divides two numbers and returns the integer value of the quotient. Unlike regular division, floor division discards the fractional part of the result, providing an integer result without rounding the number up or down. This operation is particularly useful when you want to get a whole number result without any decimal places.
Python Exponentiation (**) Operator
The exponentiation operator (**) is a powerful arithmetic operation in Python. It raises the first number (base) to the power of the second number (exponent), yielding the result of the base raised to the specified power. For example, if you have the expression 2 ** 3, it would be evaluated as 2 raised to the power of 3, which equals 8. This operator is instrumental in performing various mathematical calculations and can be used to calculate exponential growth, compound interest, and other mathematical functions involving exponents.
Python Assignment Operators
In Python, assignment operators are used to assign a value to a variable based on the value of another operand. These operators are essential for manipulating variables and data within the code. They do not produce any output or result and are mainly used for their side-effect of updating the variable on the left-hand side. The value of an assignment expression is the value that gets assigned to the variable, allowing you to store, modify, or update data during the execution of your program. Assignment operators in Python include common symbols like '=', '+=', '-=', '*=', '/=', '%=', '**=', '//=', etc., each serving a specific purpose in updating variable values.
Operator | Description | Syntax |
---|---|---|
= | x = 1 | x = 1 |
+= | x += 1 | x = x + 1 |
-= | x -= 1 | x = x - 1 |
*= | x *= 1 | x = x * 1 |
/= | x /= 1 | x = x / 1 |
%= | x %= 1 | x = x % 1 |
//= | x //= 1 | x = x // 1 |
**= | x **= 1 | x = x ** 1 |
&= | x &= 1 | x = x & 1 |
|= | x |= 1 | x = x | 1 |
^= | x ^= 1 | x = x ^ 1 |
>>= | x >>= 1 | x = x >> 1 |
<<= | x <<= 1 | x = x << 1 |
Python Comparison Operators
Comparison operators, also known as relational operators, are essential components of Python that enable you to compare two values. These operators facilitate comparisons between the contents of fields with other fields or constants. They can be used independently or in conjunction with other operators and functions in both record expressions and target field expressions. By using comparison operators, you can determine relationships between values, such as equality, inequality, greater than, less than, greater than or equal to, and less than or equal to. These comparisons are frequently used in conditional statements and loops to make decisions and control the flow of the program based on the comparison results.
Operator | Description | Syntax |
---|---|---|
== | Equal | x == y |
!= | Not equal | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
Python Equal to (==) Operator
The equal to (==) operator in Python is a comparison operator used to check if the values of two operands are equal. When the values on both sides of the operator are equal, the condition evaluates to true, and otherwise, it evaluates to false. This operator is widely used in conditional statements and expressions to compare variables or values and determine if certain conditions are met in the program. For example, x == y would be true if the values of x and y are equal.
Python Not equal to (!=) Operator
In Python, the not equal to (!=) operator is another comparison operator used to check if the values of two operands are not equal. When the values on both sides of the operator are different, the condition evaluates to true, and otherwise, it evaluates to false. This operator is useful when you want to test if two values are not identical. For example, `x != y` would be true if the values of `x` and `y` are different. It is commonly used in conditional statements and expressions to handle scenarios where two values should not be equal to each other.
Python Greater than (>) Operator
The greater than (>) operator in Python is a comparison operator used to determine if the value of the left operand is greater than the value of the right operand. When the value on the left side of the operator is indeed greater than the value on the right side, the condition evaluates to true; otherwise, it evaluates to false. This operator is commonly employed to perform conditional checks and make decisions based on the relative magnitude of values. For example, x > y would be true if the value of x is greater than the value of y.
Python Less than (<) Operator
The less than (<) operator in Python is a comparison operator used to ascertain if the value of the left operand is less than the value of the right operand. If the value on the left side of the operator is indeed smaller than the value on the right side, the condition evaluates to true; otherwise, it evaluates to false. This operator is frequently utilized in conditional statements and expressions to compare values and make decisions based on their relative magnitudes. For instance, x < y would be true if the value of x is less than the value of y.
Python Greater than or equal to (>=) Operator
The greater than or equal to (>=) operator in Python is a comparison operator used to determine if the value of the left operand is greater than or equal to the value of the right operand. If the value on the left side of the operator is greater than or equal to the value on the right side, the condition evaluates to true; otherwise, it evaluates to false. This operator is commonly used in conditional statements and expressions to make decisions based on whether one value is greater than or equal to another. For example, x >= y would be true if the value of x is greater than or equal to the value of y.
Python Less than or equal to (<=) Operator
The less than or equal to (<=) operator in Python is a comparison operator used to verify if the value of the left operand is less than or equal to the value of the right operand. When the value on the left side of the operator is less than or equal to the value on the right side, the condition evaluates to true; otherwise, it evaluates to false. This operator is commonly employed in conditional statements and expressions to make decisions based on whether one value is less than or equal to another. For instance, x <= y would be true if the value of x is less than or equal to the value of y.
Python Logical Boolean Operators
Boolean logic is a fundamental concept in computer science and mathematics, centered around three primary Boolean operators: "or," "and," and "not." These operators manipulate Boolean values (True or False) to determine the truth or falsity of logical expressions.
Operator | Description | Syntax |
---|---|---|
and | True if both the operands are true | x and y |
or | True if either of the operands is true | x or y |
not | True if operand is false | not x |
Python Bitwise Operators
Python provides bitwise operators that manipulate operands as sequences of 32 bits represented by zeroes and ones, rather than interpreting them as decimal, hexadecimal, or octal numbers. These operators efficiently perform bitwise operations on binary patterns, offering valuable capabilities for low-level manipulations. While all the binary operators are infix, the "not" operator stands as an exception. Notably, bitwise operations often exhibit superior performance compared to division, multiplication, and sometimes even addition, making them a preferred choice for optimizing computational efficiency in relevant applications.
Operator | Description | Syntax |
---|---|---|
& | Bitwise AND | x & y |
| | Bitwise OR | x | y |
^ | Bitwise NOT | x ^ y |
~ | Bitwise XOR | ~x |
<< | Bitwise right shift | x>> |
>> | Bitwise left shift | x<< |
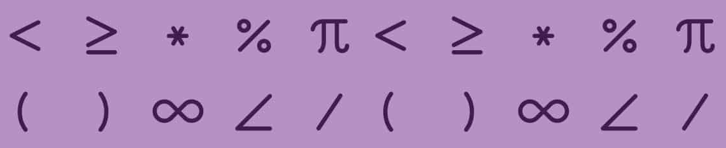
Python Membership Operators
Python provides two membership operators: "in" and "not in." These operators are used to check whether a value exists within a specified variable, such as a string, list, tuple, or set. They return a Boolean value, "True" if the value is present in the variable, and "False" if it is not found.
Operator | Description | Syntax |
---|---|---|
in | True if value is found in the sequence | x in y |
not in | True if value is not found in the sequence | x not in y |
Python Identity operators
Python provides two identity operators: "is" and "is not." These operators are used to compare objects based on their identity, which means they check if the operands refer to the same object in memory.
Operator | Description | Syntax |
---|---|---|
is | True if the operands are identical | x is y |
is not | True if the operands are not identical | x is not y |
Identity operators are particularly useful when you want to determine if two variables point to the same object, or if a variable is None. They are commonly used for type-checking, object comparison, and for testing if a variable has been assigned a value or not (e.g., checking if a variable is None).
Conclusion
Python operators are symbols that perform operations on variables or values. They include arithmetic, comparison, logical, assignment, bitwise, membership, and identity operators, allowing programmers to manipulate data, perform comparisons, and control program flow efficiently. These operators play a crucial role in Python programming, enabling a wide range of tasks, from basic arithmetic calculations to complex conditional statements and data manipulations.
- Keywords in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples