Multiple strings exist in another string : Python
In Python, to check if multiple strings exist in another string, you can use various approaches like the "in" operator, regular expressions, or custom functions. Let's explore these methods with examples:
Using "in" Operator
The "in" operator allows you to check if individual substrings exist within a given string.
Using re.findall()
For more complex pattern matching involving multiple substrings, you can use the re.findall() function from the re module.
Python any() Function
The any() function takes an iterable (such as a list, tuple, dictionary, etc.) as an argument and evaluates to true if at least one element within the iterable is true; otherwise, it returns false. If the iterable object is empty, the any() function will return False. The any() function proves particularly useful when you need to check for the presence of truthy values within an iterable, enabling efficient and concise evaluation of multiple elements.
any Vs all
- any will return True when at least one of the elements is Truthy.
- all will return True only when all the elements are Truthy.
Check if multiple strings exist in another string
In this case, we can use Python "any()" .
Here the script return "Found a match", because at least one word exists in the list.
example 2:
How to check if string contains substring from list
If your list is too long, it is better to use Python Regular Expression .
Above example return "Found a match" because "one" is exist in the list.
Check If a String Contains Multiple Keywords
You can also find a solution for this by using iteration .
Above script return "Found a match" because "one" is exist in the myList.
All matches including duplicates in a string
If you want to get all the matches including duplicates from the list:
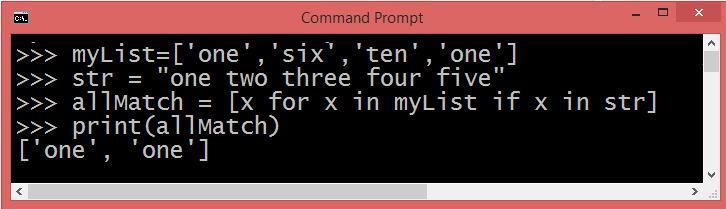
First word match in a string from list
If you want the first match with False as a default:
Above example return "one" because the word "one" is the starting word and exists in the myList also.
How to extract the first and final words from a string?
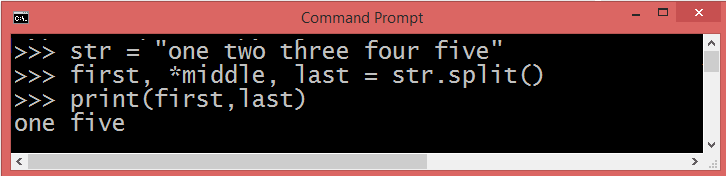
Similarly to check if all the strings from the list are found, use "all" instead of "any" .
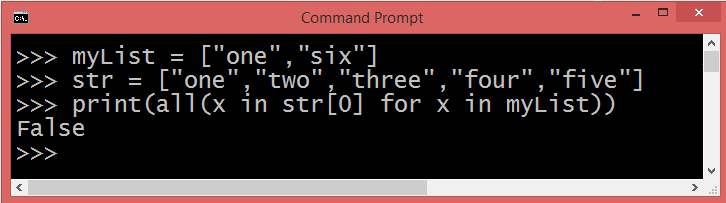
Above example return False because "six" is not in the string.
Conclusion
Each of these methods allows you to check for the existence of multiple substrings within a given string. The choice of method depends on the complexity of the patterns you need to match and your specific use case. The "in" operator is simple and straightforward for basic checks, while regular expressions offer greater flexibility for advanced pattern matching involving more complex substrings. Custom functions provide modularity and code reusability for repetitive substring checks. Choose the most suitable method based on your requirements to efficiently determine if multiple strings exist in another string in Python.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples