Python keywords list and uses
Keywords in Python are reserved words that have predefined meanings and cannot be used as variable names or identifiers in the code. These keywords are an integral part of the Python language syntax and are used to define the structure and logic of the program. Since they have predefined roles, you cannot use them for any other purpose, and attempting to do so will result in syntax errors.
Python Keywords
Keywords are used for a variety of purposes in Python, including:
- Control flow: Keywords like if, elif, and else are used to control the flow of execution in a Python program.
- Iteration: Keywords like for and while are used to iterate over sequences of data.
- Function definition: Keywords like def and lambda are used to define functions.
- Object-oriented programming: Keywords like class and self are used to implement object-oriented programming in Python.
- Exception handling: Keywords like try, except, and finally are used to handle exceptions in Python.
If you're not sure whether a word is a keyword, you can always check the Python documentation. The documentation lists all of the keywords in Python, along with their meanings and usage.
Python keywords list and uses
These keywords may vary slightly in different versions of Python, but the core set remains consistent. Let's explore each keyword in detail:
- False: A keyword representing the Boolean value "false." It is used to represent the false condition in logical expressions.
- True: A keyword representing the Boolean value "true." It is used to represent the true condition in logical expressions.
- None: A special keyword that represents the absence of a value or a null value in Python. It is often used to indicate the absence of a return value or initialize variables.
- and: A logical operator used to combine two conditions. It returns True if both conditions are true; otherwise, it returns False.
- as: This keyword is used for creating an alias (alternate name) for a module or imported module elements, making it easier to use them.
- assert: This keyword is used as a debugging aid to check if a given condition is true. If the condition is false, it raises an AssertionError exception.
- async: Introduced in Python 3.5, this keyword defines a coroutine function, which can be used with asynchronous programming.
- await: Used in conjunction with async, this keyword is used inside an asynchronous function to pause the execution and wait for an asynchronous operation to complete.
- break: This keyword is used to exit from a loop prematurely. When encountered, it immediately terminates the nearest enclosing loop.
- class: This keyword is used to define a new class, which serves as a blueprint for creating objects.
- continue: This keyword is used to skip the rest of the loop's body and jump to the next iteration.
- def: This keyword is used to define a new function with a specified name and block of code that executes when the function is called.
- del: This keyword is used to delete a reference to an object. It can be used to remove items from lists and dictionaries or delete variables.
- elif: Short for "else if," this keyword is used to specify an alternative condition to check if the previous if statement evaluates to false.
- else: This keyword is used to specify the block of code to execute when none of the preceding conditions in an if-elif chain evaluate to true.
- except: This keyword is used in exception handling to define a block of code to execute when an exception occurs.
- finally: This keyword is used in exception handling to define a block of code that always executes, whether an exception occurred or not.
- for: This keyword is used to define a loop that iterates over a sequence (e.g., list, tuple, string, etc.).
- from: This keyword is used in import statements to specify the module from which you want to import objects.
- global: This keyword is used to declare variables inside a function as global, meaning they can be accessed and modified from outside the function's scope.
- if: This keyword is used to define a conditional statement. The code block under it executes if the specified condition is true.
- import: This keyword is used to import modules or specific elements from a module into the current Python script.
- in: This keyword is used to check for membership in a sequence (e.g., a list, tuple, string, etc.).
- is: This keyword is used to check if two objects refer to the same memory location, indicating they are the same object.
- lambda: This keyword is used to create anonymous (or "nameless") functions using a concise syntax.
- nonlocal: This keyword is used to declare variables inside nested functions as non-local, allowing them to be modified in the inner function but not becoming global variables.
- not: A logical operator that negates the result of a Boolean expression.
- or: A logical operator that returns True if at least one of the conditions in the expression is true.
- pass: This keyword is a null operation; it does nothing. It is often used as a placeholder when a statement is syntactically required but no action is desired.
- raise: This keyword is used to raise exceptions explicitly.
- return: This keyword is used in functions to specify the value to be returned when the function is called.
- try: This keyword is used to define a block of code in which exceptions can occur, followed by except and/or finally blocks.
- with: This keyword is used in context managers to define a block of code that is executed in a specific context.
- while: This keyword is used to define a loop that continues as long as a given condition is true.
- yield: This keyword is used in generator functions to produce a series of values one at a time and temporarily suspend the function's execution state.
Here are some examples of how keywords are used in Python:
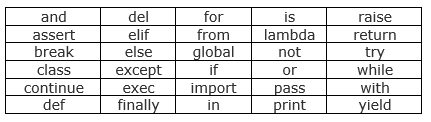
Conclusion
Remember that using any of these keywords as variable names or identifiers will result in a syntax error. Always choose meaningful and descriptive names for your variables and avoid using Python keywords as names to prevent unintended errors in your code.
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples