Installing Python Packages
Pip is a widely-used package management system in the Python ecosystem, designed to facilitate the seamless installation and management of software packages developed in the Python programming language. It provides an efficient and straightforward way for Python developers to effortlessly acquire, configure, and organize a plethora of third-party libraries and modules, ultimately enriching their projects with enhanced functionality and productivity.
On Windows:
Replace package_name with the name of the package you want to install.
On Linux:
On macOS:
How to Install Python Modules
Pip is a package management system used to install and manage software packages written in Python. Following is a step-by-step guide on how to install a package in Python using pip:
Check if pip is installed
Before you can use pip to install packages, you need to check if pip is installed in the first place. To do this, open up a terminal or command prompt and enter the following command:
This will display the version of pip installed on your system. If pip is not installed, you can download and install it from the Python website.
Find the package to install
Next, you need to find the package you want to install. You can search for packages on the Python Package Index (PyPI) website or use other sources to find packages.
Install the package
Once you've found the package you want to install, open up a terminal or command prompt and enter the following command:
Replace package_name with the name of the package you want to install. If the package has dependencies, pip will automatically download and install them as well.
Verify the installation
Once the installation is complete, you can verify it by importing the package in a Python script or interpreter. For example, if you installed the numpy package, you can open up a Python interpreter and enter the following command:
If there are no errors, the package is installed correctly.
Now you've successfully installed a package in Python using pip.
Install a specific version of a package | Python
If you want to install a specific version of a package, you can specify the version number after the package name, like this:
For example, to install version 1.19.5 of the pandas package, you would type:
Installing a List of Packages Using pip | Python
To install multiple packages, it is better using a text file. You can create a file with the names of the packages you want to install, separated by a newline character. For example, you could create a file named requirements.txt with the following contents:
Once you have created the requirements.txt file with the names of the packages you want to install, you can use the following command to install them all at once:
This command will read the requirements.txt file and install all the packages listed in it.
Note that the names of the packages in the text file should be the exact same as the package names listed on PyPI. Additionally, you can specify the version number of each package in the text file by including the version number after the package name, separated by a space. For example:
This will ensure that the specified version of each package is installed.
What is PIP?
Pip is a package management system used to install and manage software packages written in Python. It simplifies the process of installing and managing third-party Python packages and their dependencies. With pip, you can easily install, upgrade, and uninstall Python packages from the PyPI (Python Package Index), a repository of software packages for Python.
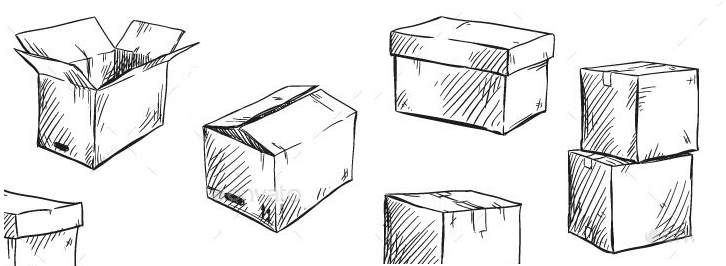
Pip is included with Python versions 2.7.9 and later (Python 3.4 and later), so you don't need to install it separately if you have a recent version of Python installed. However, if you have an older version of Python or if you need a more recent version of pip, you can install it manually.
Pip is a command-line tool, which means that you use it by typing commands into a terminal or command prompt. There are many pip commands that you can use to manage Python packages, including installing, upgrading, uninstalling, searching, and listing packages.
Commonly used pip commands
Uninstalling packages:
This command uninstalls the specified package and any of its dependencies.
Upgrading packages:
This command upgrades the specified package to the latest version.
Listing installed packages:
This command lists all the installed packages and their versions.
Searching for packages:
This command searches the PyPI repository for packages that match the given name.
Creating a requirements file:
This command generates a requirements file requirements.txt that contains a list of all installed packages and their versions.
Installing packages from a requirements file:
This command installs all the packages listed in the requirements.txt file.
You can learn about them by typing pip --help in your terminal or by visiting the official pip documentation at https://pip.pypa.io/en/stable/.
Conclusion
Installing Python packages is a crucial task to extend the functionality of Python. It can be done using package managers like pip or conda, which fetch and install packages from online repositories, making it convenient to add external libraries and modules to Python projects for enhanced capabilities and productivity.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples