How to Get a Substring From a String in Python
In Python, strings are sequences of bytes that represent Unicode characters. Accessing individual characters within a string can be achieved using array-like indexing. Similar to array data types, where items are associated with an index number, each character in a string is also associated with an index number, starting from 0.
Python substring
Substring-specific methods like substring() or substr() are not available. Instead, we utilize slice syntax to extract portions of existing strings. Python's slicing is a highly efficient method for systematically accessing specific segments of data. The use of colons (:) in subscript notation allows us to employ slice notation, which consists of the start, stop, and step arguments, enabling flexible string manipulation. It follows this template:
- Parameters are enclosed in the square brackets.
- Parameters are separated by colon.
- start - Starting index of string, Default is 0.
- end - End index of string which is not inclusive .
- step - An integer number specifying the step of the slicing. Default is 1.
How Indexing Works
Indexing for strings allows the use of positive or negative numbers. Positive numbers represent positions from the start of the string, while negative numbers count positions from the end of the string. When using negative numbers for start and stop, counting begins from the last character, and for the optional step argument, the index is decremented accordingly. This flexibility in indexing provides convenient ways to navigate and extract substrings from both ends of the string.
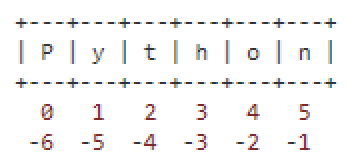
Python substring examples
Get all characters of the stringEnd index only
Start index only
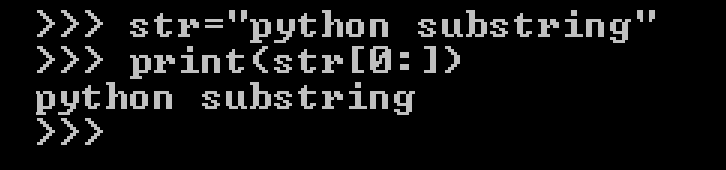
No Indexes specified
How to get the first part from a string
Get last part from a string
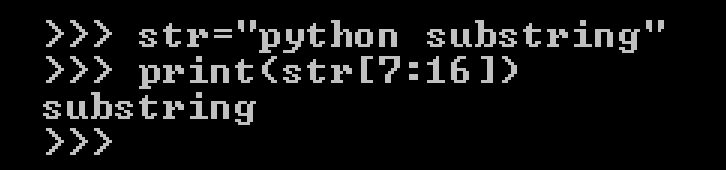
Get first character from a string
Get last character from a string
Substring from right side of the string
Python allows obtaining substrings from the right side of the string using negative indexing. A negative index indicates counting from the end of the string, from right to left, rather than from the beginning. For instance, index [-1] corresponds to the last character of the string, [-2] represents the second-to-last character, and so forth. This feature enables efficient access to characters starting from the end of the string.
How to get the last 9 character from Python string
Get a substring which contains all characters except the last 10 characters
Step in Python substring
The third parameter of slicing specifies the step, which determines the number of characters to move forward after the first character is retrieved from the string. By default, Python sets the step to 1, meaning that every character between the start and stop index numbers will be retrieved. However, you can modify the step value to skip characters and retrieve every nth character within the specified range. For example, using a step of 2 will extract every other character, and using a step of -1 will reverse the string. This flexibility in step allows for versatile string manipulation when slicing.
Add step 1
Here, we get the same results by including a third parameter with a step of 1.
Get every other character from a python string
Change step to 2
Here you can see, you get the every other character from python string .
Change step to 3
Reverse a string in Python
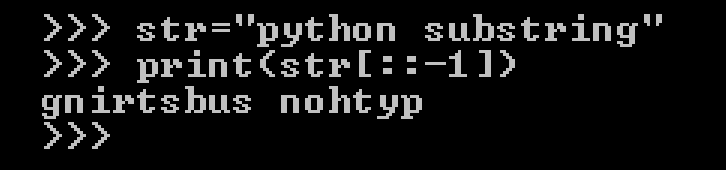
Conclusion
Python's substring slicing provides a flexible way to manipulate strings and efficiently access specific portions of data within a string. Keep in mind that slicing in Python does not modify the original string but returns a new substring as a result.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples