Python Timestamp Examples
A Python timestamp is a representation of a point in time as the number of seconds that have elapsed since January 1, 1970, at 00:00:00 UTC. This date and time is known as the "epoch", and serves as a reference point for all timestamps. In Python, timestamps are typically represented as float values, with precision to the microsecond.
Get the curent timestamp in Python
You can obtain the current timestamp in Python using the time module's time() function.
Above code will output the current timestamp as a float value. To format the timestamp as a human-readable string, you can use the datetime module
Convert timestamp to a datetime
You can convert a timestamp to a datetime object in Python using the datetime module's datetime class and the fromtimestamp method. The fromtimestamp method takes a timestamp as input and returns a corresponding datetime object.
This will output the current date and time as a datetime object, which can be formatted as a string using the strftime method.
Above code will output the current date and time as a string in the format YYYY-MM-DD HH:MM:SS. The strftime method takes a format string as input and returns a formatted string representation of the datetime object. You can customize the format string to get the desired output.
Convert datetime to timestamp
You can convert a datetime object to a timestamp in Python using the timestamp method of the datetime class. The timestamp method returns the timestamp as a float representing the number of seconds since the epoch (January 1, 1970, at 00:00:00 UTC).
Above code will output the current date and time as a timestamp in seconds as a floating-point number. Note that the datetime.now() method is used to get the current date and time as a datetime object, but you can also use any other datetime object as input.
Convert timestamp to a datetime using calendar Module
The calendar module in Python also provides functionality to work with timestamps. One way to use the calendar module to convert a timestamp to a datetime object is by using the gmtime function. This function takes a timestamp as input and returns a corresponding time tuple, which can be passed to the datetime class constructor to create a datetime object.
Above code will output the current date and time as a datetime object, which can be formatted as a string using the strftime method, as shown in the previous section.
Convert Timestamp to String
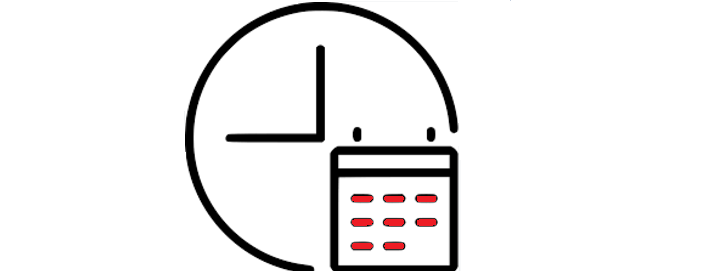
Convert a timestamp to a string in Python, you first need to convert the timestamp to a datetime object, and then use the strftime method to format the datetime object as a string.
Above code will output the current date and time as a string in the format YYYY-MM-DD HH:MM:SS. The strftime method takes a format string as input and returns a formatted string representation of the datetime object. You can customize the format string to get the desired output.
Get Timestamp in Milliseconds
In order to get the timestamp in milliseconds in Python, you need to multiply the value returned by the time.time() function by 1000. This will give you the timestamp in seconds multiplied by 1000, which represents the number of milliseconds since the epoch (January 1, 1970, at 00:00:00 UTC).
Above code will output the current timestamp in milliseconds as an integer value. Note that the value is rounded to the nearest integer using the round function, and then converted to an integer using the int function.
Get the Current Date and Time using timestamp
To get the current date and time in Python using a timestamp, you can use the time module to get the current time as a timestamp, and then use the datetime module to convert the timestamp to a datetime object.
Above code will output the current date and time as a datetime object. You can then use the strftime method to format the datetime object as a string, as I mentioned in my previous answers.
Conclusion
A timestamp represents a point in time, usually measured in seconds since the Unix Epoch (January 1, 1970, at 00:00:00 UTC). Python provides various ways to work with timestamps, such as using the time module to access time-related functions or the datetime module for more advanced date and time operations, making it easy to manage and manipulate time-related data in Python applications.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)