Basics of Memory Management in Python
Memory management in Python is the process of allocating and deallocating memory for objects in Python. Python uses a garbage collector to manage memory. The garbage collector automatically deallocates memory that is no longer being used by an object.
There are two types of memory in Python:
- Stack memory: Stack memory is used to store local variables and function call frames. Stack memory is allocated when a function is called and deallocated when the function returns.
- Heap memory: Heap memory is used to store objects that are created by the programmer. Heap memory is allocated and deallocated by the garbage collector.
When an object is created in Python, it is allocated memory on the heap. The object's memory is not deallocated until it is no longer being used. The garbage collector determines when an object is no longer being used by tracking references to the object. If there are no references to an object, the garbage collector will deallocate the object's memory.
Python Memory Manager
Python Memory Manager is responsible for the allocation and deallocation of memory during the execution of Python programs. It incorporates several strategies and techniques to optimize memory usage and ensure efficient memory management.
Object Allocation and Deallocation
When you create objects in Python, such as variables, lists, dictionaries, or custom objects, the Memory Manager dynamically allocates memory to store those objects. The Memory Manager keeps track of the reference count for each object using the reference counting mechanism. When an object's reference count drops to zero, indicating that there are no more references to the object, the Memory Manager automatically deallocates the memory occupied by that object, making it available for reuse.
Memory Pools and Small Object Optimization
Python uses memory pools to manage the allocation of small objects. Instead of requesting memory from the operating system for each small object, Python pre-allocates memory blocks and divides them into smaller chunks for reuse. This strategy, known as small object optimization, reduces the overhead of frequent memory allocations and deallocations for small objects, improving memory allocation efficiency.
Garbage Collection
While reference counting is effective in most cases, it may not handle cyclic references, where objects refer to each other in a circular manner, leading to memory leaks. To address this issue, Python employs a garbage collector. The garbage collector identifies cyclically referenced objects that are no longer reachable and clears their memory, ensuring efficient memory management even in complex scenarios.
Memory Profiling
Python provides tools and modules for memory profiling and monitoring memory usage in your programs. The sys.getsizeof() function allows you to measure the size of individual objects, and the gc module provides functionalities to control and analyze the garbage collector.
Let's look at a simple example to illustrate the Memory Manager in Python:
In this example, we use sys.getsizeof() to measure the memory usage of lists 'a', 'b', and the concatenated list 'c'. We can observe that 'c' takes more memory than the sum of 'a' and 'b', as Python creates a new list for the concatenation. This illustrates how the Memory Manager handles memory allocation and deallocation in Python.
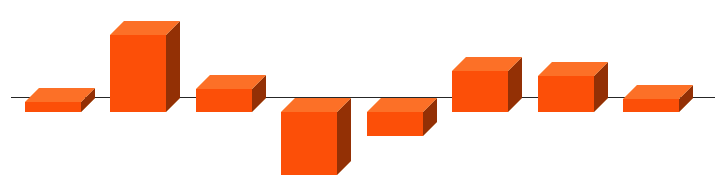
Memory management in Python is a critical aspect of how the language handles the allocation and deallocation of memory resources during the execution of a program. Python uses an automatic memory management system that incorporates features like reference counting and a garbage collector to efficiently manage memory and prevent memory leaks.
Memory Leak

A memory leak is a situation where an object is no longer in use but the memory for the object is not deallocated. Memory leaks can cause your program to run out of memory and crash.
To avoid memory leaks, you should make sure that you explicitly delete objects when you are no longer using them. You can also use the weakref module to create weak references to objects. Weak references are not tracked by the garbage collector, so they will be garbage collected even if they are still being referenced.
Conclusion
While Python's memory management is generally efficient and transparent to developers, it is essential to be mindful of certain scenarios, such as circular references or large data structures, which can impact memory consumption. By following best practices and using appropriate data structures, developers can optimize memory usage and ensure smooth and efficient execution of Python programs.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples