Python 2D Arrays
A 2D array, also known as a two-dimensional array or a matrix, is a data structure that represents a collection of elements arranged in a grid of rows and columns. It is essentially an array of arrays, where each element of the outer array is itself an array of elements. Each element in a 2D array is identified by two indices: the row index and the column index.
2D arrays are commonly used in computer programming to represent tables of data, such as spreadsheets or game boards. For example, a chess board can be represented as an 8x8 matrix, where each element in the matrix represents a square on the board. Similarly, an image can be represented as a 2D array of pixels, where each pixel is represented by a set of values that determine its color and brightness.
The size of a 2D array is determined by the number of rows and columns it contains. For example, a 3x4 matrix contains 3 rows and 4 columns, for a total of 12 elements. The elements in a 2D array can be of any data type, such as integers, floating-point numbers, or strings.
Python 2D Array | Examples
In Python, a 2D array, also known as a matrix, is a collection of data arranged in a grid of rows and columns. A 2D array can be represented as a list of lists, where each list represents a row of the matrix. Each element in the matrix is accessed using its row and column indices.
Following is an example of creating a 2D array in Python:
In the above example, the matrix is a 3x3 grid with integers from 1 to 9. Each row of the matrix is represented as a list.
How to create a 2D array in Python?
Following is an example of how to create a 2D array in Python:
In the above example, first create a 2D array of size 3x4 with all elements initialized to 0. Use a list comprehension to create the outer list of rows, and another list comprehension to create each inner list of columns. Next, fill the matrix with values using a nested for loop. For each row i and column j, set the value of matrix[i][j] to the sum of i and j. Finally, print the matrix using another nested for loop. Print each element of the matrix followed by a space, and then print a newline character after each row.
This is a 3x4 matrix where each element is the sum of its row and column indices.
How to access elements in a 2D array in Python
In Python, you can access elements in a 2D array using their row and column indices. Following is an example of how to access elements in a 2D array:
Access a Single Element in 2d array
Access an Inner Array in 2d array
In a 2D array, accessing an inner array can be done by specifying the index of the outer array to get the inner array, and then specifying the index of the inner array to get the element you want.
For example, suppose you have the following 2D array. To access the inner array at index 1 (which contains [4, 5, 6]), you would use the following code:
How to update elements in a 2D array in Python
In Python, you can update elements in a 2D array using their row and column indices. Following is an example of how to update elements in a 2D array:
Updating a Single Element in 2d array
Updating an Inner Array in 2d array
To update an inner array in a 2D array in Python, you can use the indexing notation with the row index of the inner array and assign a new list to it.
How to delete elements from a 2D array in Python
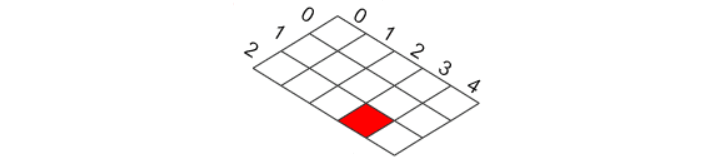
In Python, you can delete elements from a 2D array by using the del keyword to remove the entire row or column containing the element you want to delete. Following is an example of how to delete elements from a 2D array:
Deleting an Inner Array in 2d array
Deleting a Single Element in 2d array
To delete a single element in a 2D array in Python, you can use the del statement with the indexing notation with the row and column indices of the element you want to delete.
How to iterate over a 2D array in Python
In Python, you can iterate over a 2D array using nested for loops. Following is an example of how to iterate over a 2D array:
How to find the length of a 2D array in Python
You can find the length of a 2D array by using the len() function. The length of a 2D array is the number of rows in the array.
How to transpose a 2D array in Python
You can transpose a 2D array using the built-in zip() function. The zip() function takes one or more sequences as input and returns an iterator of tuples where the first element in each tuple comes from the first sequence, the second element comes from the second sequence, and so on.
How to convert a 2D array into a 1D array in Python
You can convert a 2D array into a 1D array using the numpy library. The numpy library provides a flatten() function that can be used to convert a 2D array into a 1D array.
How to concatenate two 2D arrays in Python
You can concatenate two 2D arrays using the numpy library's concatenate() function. This function allows us to concatenate two arrays along a specified axis.
Following is an example of how to concatenate two 2D arrays using the numpy library:
How to sort a 2D array in Python
You can sort a 2D array using the numpy library's sort() function. This function allows us to sort the elements of an array along a specified axis.
This shows that you can sort a 2D array in Python using the numpy library's sort() function. Note that you need to specify the axis along which to sort the array. In this example, sorted the matrix along the rows, so the resulting matrix has the smallest elements in the first row and the largest elements in the last row.
How to search for an element in a 2D array in Python
To search for an element in a 2D array in Python, you can use a nested loop to iterate over each element in the array and check if the element matches the target value.
How to compare two 2D arrays in Python
To compare two 2D arrays in Python, you can iterate over each element in both arrays and compare them.
How to perform arithmetic operations on two 2D arrays
Performing arithmetic operations on two 2D arrays in Python involves performing the operation on corresponding elements of both arrays. Following are some examples:
Add the two 2D arrays
Subtract the two 2D arrays
Multiply the two arrays
Divide the two 2D arrays
Above examples show how you can perform subtraction, multiplication, and division on two 2D arrays in Python. The approach is similar to that used for addition: we use nested loops to iterate over each element in both arrays and perform the appropriate operation.
How to initialize a 2D array with default values in Python
To initialize a 2D array with default values in Python, you can use nested loops to create a new array and assign the default value to each element.
In the above example, create a 2D array with 3 rows and 4 columns and initialize each element to the default value 0 using nested loops. Then print the initialized array using another loop.
Above example shows that you can initialize a 2D array with default values in Python by using nested loops to create a new array and assign the default value to each element.
You can replace the default value of 0 with any other value you want to initialize the array with.
Conclusion
A 2D array is a data structure that organizes elements in a grid-like fashion, with rows and columns forming a matrix. It is implemented using nested lists or arrays, providing a convenient way to store and manipulate data in a tabular format, facilitating various applications such as image processing, board games, and numerical computations.