Python Classes/Objects
A class is a blueprint for creating objects, which are instances of the class. A class defines a set of attributes and methods that can be used to create objects with the desired characteristics and behaviors. The purpose of a class in Python (and in object-oriented programming in general) is to provide a blueprint for creating objects with specific attributes and behaviors.
Classes are used to encapsulate related data and functions into a single unit, making it easier to manage and maintain code. They allow you to create custom data types that can be used throughout your program, and can be used to model real-world objects or abstract concepts. One of the main benefits of using classes is code reuse. Once a class is defined, you can create multiple instances of that class, each with their own values for the attributes defined in the class. This can be useful when you need to create multiple objects with similar properties or behaviors.
In addition, classes promote abstraction and modularity in programming. By defining a class that represents a concept or object, you can separate its implementation from the rest of the program. This can make the code more flexible and easier to modify in the future, as changes to the implementation of the class will not affect the rest of the program. Also, classes allow for inheritance, which is a mechanism for creating new classes based on existing ones. Inheritance allows you to reuse code and add new features to a class without modifying its original definition. This can save time and reduce errors in code development.
Define a class in Python
To define a class in Python, you use the class keyword followed by the name of the class. Following is the basic syntax:
Inside the class definition, you can define attributes, methods, and other class-related properties.
Following is an example of defining a class in Python:
In the above example, define a class Employee with two attributes, name and id. The __init__() method is a constructor that is called when the object is created and is used to initialize the object's attributes.
__init__ method (Python Constructor)
The __init__() method is a special method in Python classes that is called when an object of the class is created. It is commonly referred to as the constructor method, as it initializes the object's attributes when it is first created.
The __init__() method is defined with the following syntax:
In the baove code, self refers to the instance of the class being created, and parameter1, parameter2, etc. are the parameters passed to the constructor when the object is created.
The __init__() method can be used to initialize any attributes of the object, which are accessed using the self keyword.
In the above example, the __init__() method takes two parameters, name and id, and initializes two attributes of the object, self.name and self.id, with those values.
Create an instance of a class in Python
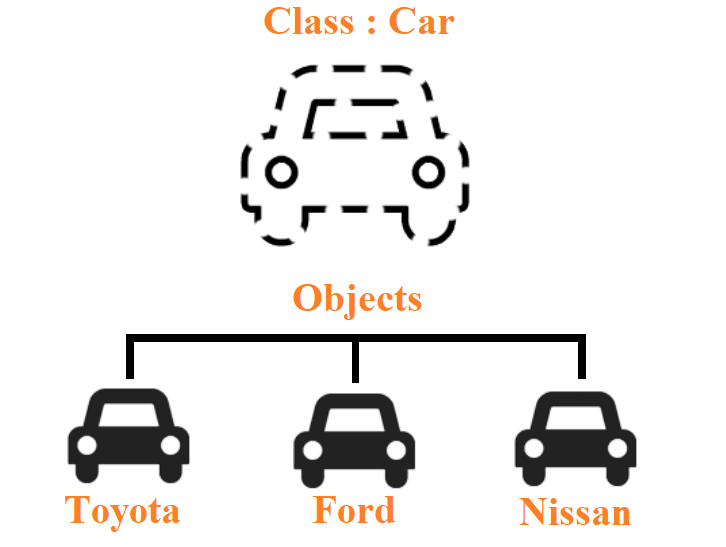
To create an instance of a class in Python, you simply call the class constructor, which is defined using the __init__() method. Following is an example of creating an instance of a class:
In the above example, create an instance Employee1 of the Employee class and pass in two arguments, "Bill" and 101, to the constructor method. This creates a new Person object with the name attribute set to "Bill" and the is attribute set to 101.
To create an instance of Employee, simply call its constructor with the necessary arguments. This creates an instance of Employee and assigns it to the variable Employee1.
Once you have an instance of Employee, you can access its attributes using dot notation, like so:
In the above example, you access the Employee1.name attribute of Employee using dot notation, and print its value to the console.
Attributes and methods of a Python class
Attributes and methods are two fundamental components of a Python class.
Class Attributes
Attributes are variables that store data associated with an object. They represent the state of the object, and their values can be accessed and modified using dot notation. Attributes are defined within the class definition and are initialized using the constructor method. They can be either instance attributes, which are unique to each instance of the class, or class attributes, which are shared by all instances of the class.
Following is an example of defining attributes in a Python class:
Class Methods
Methods are functions that are defined within a class and are used to perform specific actions on an object. They can be used to manipulate the state of the object or to perform operations on its attributes. Methods can be defined to take parameters and can return values.
Following is an example of defining a method in a Python class:
In the above example, define a class Employee with an instance method emp_details(). This method takes no parameters and prints out a message using the name and id attributes of the object.
The self parameter
The self is a special parameter that is used to refer to the instance of a class. When a method is called on an object, self refers to that particular instance of the class, allowing the method to access and manipulate the object's attributes.
When defining a method in a class, the self parameter is always the first parameter of the method definition.
In the above code, my_method() is a method of the MyClass class that takes two arguments, arg1 and arg2, as well as the self parameter. When my_method() is called on an instance of the MyClass class, the self parameter will automatically be set to that instance.
For example, if you create an instance of MyClass like so:
You can then call my_method() on my_object like so:
In the above case, the self parameter of my_method() will automatically be set to my_object, allowing the method to access and manipulate my_object's attributes.
Conclusion
A Python class is a blueprint for creating objects that encapsulate data and behavior. It allows you to define attributes and methods that represent the characteristics and actions of the objects. To use a Python class, you instantiate it to create individual objects, and then you can access and manipulate their attributes and call their methods to perform specific tasks.