How To Use the Python Map Function
Python map() is a useful tool for simplifying code when you need to apply a function to each element of an iterable object. It is a built-in higher-order function that applies a given function to each item of an iterable and returns an iterable with the results. It takes two arguments: the first argument is the function to be applied to each item, and the second argument is the iterable object.
Python map() returns an iterator object that can be converted to other iterable objects like list, tuple, and set. It is commonly used when you want to apply the same function to each element of a list or another iterable object. In Python 3, map() returns an iterator object instead of a list, so you need to convert it to a list or another iterable object to see the output.
Syntax:Where function is the function to be applied to each item of iterable. The function argument can be a built-in function or a user-defined function. iterable is the iterable object that you want to apply the function to.
How does the Map function work
The map() function takes two arguments: a function object and an iterable. The iterable can be any object that can be looped over, such as a list, tuple, or string. map() applies the function to each element of the iterable in turn, and returns an iterator that contains the transformed values.
The function that is passed as the first argument to map() must take one argument and return a value. This function is applied to each element of the iterable in turn, and the resulting transformed value is yielded by the iterator. The returned iterator can be looped over using a for loop or consumed using other iterator methods like next().
The map() function is lazy, which means that it only produces values on demand, when you request them through iteration. This is what makes map() an efficient way to apply a function to an iterable when you only need to process the transformed values one at a time. The transformed values are produced in the same order as the original iterable, which means that the first transformed value in the iterator corresponds to the first element in the iterable, the second transformed value corresponds to the second element, and so on.
Python map() function | Examples
Following is an example of how you can use the map() function to square each number in a list:
In the example above, define a function called square() that takes a number as input and returns the square of that number. Then create a list of numbers and use the map() function to apply the square() function to each number in the list. The result is a new iterable object (in this case, a map object) that contains the squared numbers. Then convert this object to a list using the list() function and print the result.
Lambda function with map()
You can also use a lambda function with the map() function to avoid defining a separate function.
In the above example, use a lambda function instead of defining a separate square() function. The lambda function takes a number as input and returns the square of that number. Then use the map() function with the lambda function to square each number in the list, and print the result as a list.
Map function with multiple arguments
The map() function can also be used with multiple iterables and functions. Following is an example of how you can use map() with two lists and a function that adds two numbers:
In the above example, define a function called add() that takes two numbers as input and returns their sum. Then create two lists of numbers and use the map() function with the add() function to add the corresponding elements of each list. The result is a new iterable object that contains the sums of the corresponding elements. Then convert this object to a list using the list() function and print the result.
Using if statement with map()
You can use an if statement with the map() function to apply a function to only those items in an iterable that meet a certain condition.
Following is an example of how you can use an if statement with map() to square only the even numbers in a list:
In the above example, first define a function called square() that takes a number as input and returns the square of that number. Then create a list of numbers and use the filter() function with a lambda function to filter only the even numbers in the list. Then use the map() function with the square() function to square each of the even numbers in the filtered list. Finally, convert the result to a list using the list() function and print the squared even numbers.
Map with other iterable objects besides lists in Python
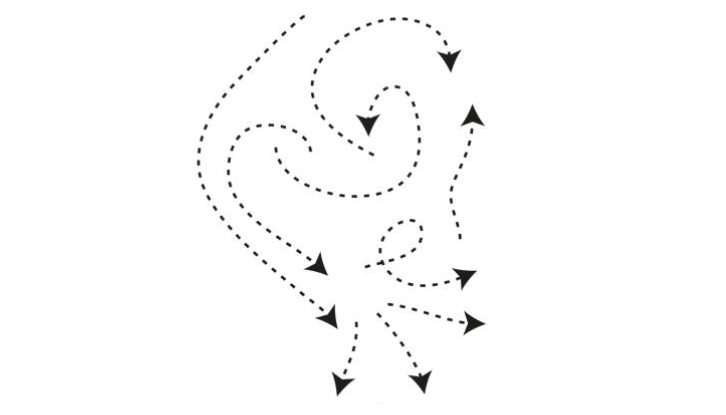
You can use map() with other iterable objects besides lists in Python. map() can be used with any iterable object that can be looped over, such as tuples, strings, sets, dictionaries, and even user-defined iterables like generators.
Python map() with a tuple
Python map() with a string
Python map() with a dictionary
Python map() with a generator
Above program generates the first 5 numbers in the Fibonacci sequence using the fibonacci() function and then squares each number using the square() function and map() function. Note that the fibonacci_iterator is an iterator object, so it can only be iterated through once. If you wanted to iterate through the squared Fibonacci numbers again, we would need to generate a new iterator object using map() and fibonacci().
How to map() and filter()
In Python, map() and filter() are both built-in functions that operate on iterables and return iterator objects. The map() function takes a function and an iterable as arguments, applies the function to each element of the iterable, and returns an iterator that contains the transformed values. The filter() function takes a function and an iterable as arguments, applies the function to each element of the iterable, and returns an iterator that contains only the elements for which the function returns True.
Following is an example of using map() and filter() together:
In the above example, define two functions square() and is_even() that take one argument and return a value. Then create a list of numbers and apply map() to square each number, and filter() to keep only the even numbers. Finally, combine map() and filter() to square only the even numbers in the list. Loop over the resulting iterators and print out the values one by one.
Note that you can chain map() and filter() calls to create more complex transformations of iterables. You can also use list comprehension to achieve the same result.
Conclusion:
The map() function in Python applies a given function to each element of an iterable and returns an iterator that contains the transformed values. The resulting iterator can be consumed using a for loop or other iterator methods, and the transformed values are produced lazily one at a time as they are requested through iteration.