How to Implement a Python Stack
A stack in Python can be thought of as a collection of elements, where elements can only be added or removed from one end of the collection, known as the top of the stack. The two primary operations that can be performed on a stack are "push" and "pop". When an element is added to the top of the stack, this is known as a "push" operation. When an element is removed from the top of the stack, this is known as a "pop" operation.
In addition to the push and pop operations, stacks also have a "peek" operation, which allows you to look at the topmost element without removing it. You can also check if a stack is empty or not, and clear the stack completely.
Python stacks are often used in algorithm design, as they provide a simple way to store and retrieve data in a last-in-first-out order. They can also be used in solving problems that require backtracking, such as depth-first search algorithms.
LIFO Principle of Stack
The LIFO principle is the fundamental property of a stack data structure. In a stack, elements are added and removed from only one end, known as the top of the stack. The last element added to the stack is the first one to be removed, and this is known as the last-in-first-out (LIFO) principle.
This means that when a new element is added to the stack, it is placed on top of the existing elements, and becomes the new top of the stack. When an element is removed from the stack, it is always the element that is currently at the top of the stack.
The LIFO principle is important because it allows stacks to be used in a variety of applications, particularly in algorithms and data processing where data must be processed in a particular order. For example, if you need to process a series of data items in reverse order of their occurrence, you can use a stack to store the items in the order they are received, and then retrieve them in reverse order by popping them off the stack.
The LIFO principle is also important for memory management in computer programs, where stacks are often used to manage the call stack for functions and procedures. Each time a function is called, its return address is pushed onto the stack, and when the function completes, the address is popped off the stack to return control to the calling function.
Implement a stack using a list data structure
In Python, you can implement a stack using a list data structure. Following is a simple implementation:
In the above example, create a new stack object, push three elements onto the stack, and then use the peek and pop methods to retrieve the top elements of the stack. Also use the is_empty method to check if the stack is empty after popping all the elements.
Let's go through each method one by one:
- __init__: This is the constructor method that initializes an empty list to store the stack elements.
- is_empty: This method returns True if the stack is empty, and False otherwise.
- push: This method adds an element to the top of the stack by appending it to the end of the list.
- pop: This method removes and returns the top element from the stack. It raises an IndexError if the stack is empty.
- peek: This method returns the top element of the stack without removing it. It raises an IndexError if the stack is empty.
Stack Implementation using collections.deque
You can implement a stack using the deque class from the collections module. deque stands for "double-ended queue", and it provides a way to add and remove elements from both ends of the queue, making it ideal for implementing a stack.
Following is an example implementation:
In the above implementation, define a Stack class with the same four methods as the previous implementation: __init__, is_empty, push, pop, and peek. The only difference is that we initialize the items attribute as a deque object instead of a list.
The is_empty, push, pop, and peek methods work the same way as in the previous implementation. The push method adds an element to the top of the stack using the append() method of the deque object, and the pop method removes and returns the top element using the pop() method of the deque object.
Following is an example of how to use this implementation of the stack:
In the above example, create a new stack object, push three elements onto the stack, and then use the peek and pop methods to retrieve the top elements of the stack. Also use the is_empty method to check if the stack is empty after popping all the elements.
Stack Implementation using a singly linked list
A stack can also be implemented using a singly linked list data structure. In this implementation, each node in the linked list contains the data element and a reference to the next node in the stack. The top of the stack is represented by the head node of the linked list.
Following is an example implementation of a stack using a singly linked list in Python:
In the code implementation, the Node class represents a node in the singly linked list, and the Stack class represents the stack data structure. The is_empty method checks whether the stack is empty by checking whether the head node is None. The push method adds a new node to the top of the stack by creating a new Node object and setting its next reference to the current head node. The pop method removes and returns the top element of the stack by setting the head node to the next node in the linked list and returning the data of the popped node. The peek method returns the top element of the stack without removing it by returning the data of the head node.
This implementation of a stack using a singly linked list has a time complexity of O(1) for push, pop, and peek operations, and does not have a fixed size limit like an array-based implementation.
Python Stacks and Threading
A stack can be useful for managing resources in a threaded program. When multiple threads are running in a program, they may need to share resources, such as memory or input/output devices. If two threads attempt to access the same resource at the same time, it can lead to race conditions, deadlocks, or other synchronization problems.
One way to avoid these problems is to use a stack to manage access to shared resources. Each thread can push a resource onto the stack when it needs to use it, and pop it off the stack when it's finished. By doing this, each thread is guaranteed exclusive access to the resource while it's on the top of the stack, and other threads cannot access it until it's popped off the stack.
Following is an example implementation of a stack-based resource manager in Python:
In this implementation, the ResourceManager class uses a stack to manage resources. The push_resource method pushes a resource onto the top of the stack, while the pop_resource method pops the top resource off the stack. The lock object is used to ensure that only one thread can access the stack at a time.
Following is an example of how to use the ResourceManager class:
In this example, create a ResourceManager object and push 10 resources onto the stack. Then create 5 worker threads, each of which pops a resource off the stack, uses it, and then pushes it back onto the stack. Finally, wait for all the worker threads to finish using the join method.
By using a stack-based resource manager, you can ensure that each thread has exclusive access to a resource while it's using it, and avoid synchronization problems caused by multiple threads accessing the same resource at the same time.
What is the maximum size of a stack in Python
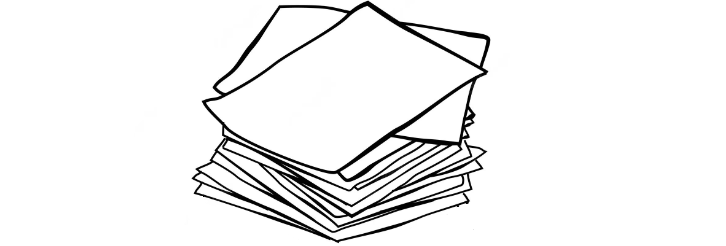
The maximum size of a stack in Python depends on the amount of memory available in the system. In Python, you can create a stack using a list, and the size of the list is limited only by the amount of memory available in the system.
However, it's important to keep in mind that as the size of the stack increases, so does the amount of memory required to store it. If you attempt to push too many elements onto the stack, you may run out of memory and cause a MemoryError.
In practice, the maximum size of a stack depends on the available memory and the specific requirements of your program. If you need to store a very large number of elements in a stack, you may need to use a more memory-efficient data structure, such as a linked list or a tree.
Applications of Stack Data Structure
The stack data structure has many practical applications in computer science and software development. Here are some common applications of the stack:
- Function call stack: When a program calls a function, the CPU creates a new frame on the call stack to store the local variables and return address of the function. When the function returns, the CPU pops the frame off the stack and returns control to the calling function.
- Expression evaluation: The stack is used to evaluate expressions in programming languages. Operators and operands are pushed onto the stack, and when an operator is encountered, the top operands are popped off the stack and the result is pushed back on.
- Undo/Redo functionality: Many applications, such as text editors or graphics software, use stacks to implement undo and redo functionality. When a user performs an action, the application pushes the action onto the undo stack. If the user wants to undo the action, the application pops the action off the undo stack and pushes it onto the redo stack.
- Browser history: Web browsers use a stack to implement the back button functionality. When a user visits a new page, the URL of the current page is pushed onto the stack. When the user clicks the back button, the browser pops the previous URL off the stack and navigates to that page.
- Recursive algorithms: Recursive algorithms, such as tree traversal or searching algorithms, use the call stack to keep track of the recursive calls. Each recursive call pushes a new frame onto the stack, and when the base case is reached, the frames are popped off the stack in reverse order.
Time complexity of push and pop operations in a stack
The time complexity of the push and pop operations in a stack implemented using a list is O(1), which is constant time.
The reason for this is that a list in Python is implemented as a dynamic array, which means that adding or removing an element from the end of the list (which is where the stack's top is located) takes constant time, regardless of the size of the list.
When you push an element onto the stack, the append() method is used to add it to the end of the list. Since append() takes constant time, the push operation also takes constant time.
When you pop an element from the stack, the pop() method is used to remove and return the last element of the list. Since pop() also takes constant time, the pop operation also takes constant time.
It's worth noting that the time complexity of the peek operation, which returns the top element of the stack without removing it, is also O(1) since it only involves accessing the last element of the list.
Conclusion
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. It allows elements to be added and removed only from the top, making it suitable for managing function calls, parsing expressions, and handling undo/redo operations efficiently.