How to Perform Floor Division in Python
Python's floor function is a built-in function that is used to round a given number down to the nearest integer that is less than or equal to the given number. The function is part of the math module in Python and can be called using the syntax math.floor(x), where x is the number to be rounded down.
Consider the following code:
In the above code, first import the math module using the import statement. Then define a variable x and assign it the value 5.8. Next, call the math.floor function with x as its argument and assign the result to a variable y. Finally, print the value of y using the print statement.
The output of this code will be: 5As you can see, the floor function has rounded down the value of x to the nearest integer that is less than or equal to x, which is 5.
How does the floor function handle negative numbers?
The floor function in Python handles negative numbers by rounding them towards negative infinity. In other words, if you pass a negative number to the floor function, it will round it down to the nearest integer that is less than or equal to the input number.
Consider the following code:
In the above example, the input value -4.5 is rounded down to -5 by the floor function, which rounds towards negative infinity.
It's important to note that the floor function always rounds down, regardless of the sign of the input value. This means that if you pass a positive number to the floor function, it will round it down to the nearest integer that is less than or equal to the input number, and if you pass a negative number, it will round it down to the nearest integer that is less than or equal to the input number (in this case, towards negative infinity).
Floor function with floating-point numbers
It is important to note that the floor function always returns an integer value, even if the input value is a floating-point number.
In the above case, the floor function has rounded down the value of x to the nearest integer that is less than or equal to x, which is 3.
What is the purpose of the floor function in Python
The purpose of the floor function in Python is to round a given number down to the nearest integer that is less than or equal to the given number. This function is useful in situations where you need to work with integer values, such as when dealing with discrete data or when performing calculations that require integer input.
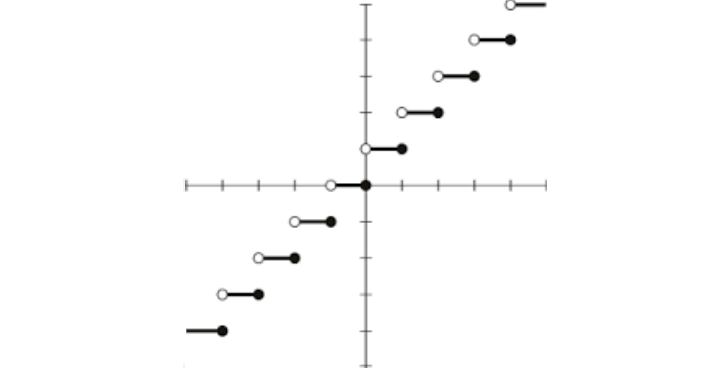
One common use case for the floor function is in financial calculations. When working with money, it is often necessary to round values down to the nearest penny, dollar, or other currency unit. The floor function can be used to accomplish this by rounding down to the nearest integer value. For example, if you have a floating-point value representing a price and you want to round it down to the nearest dollar, you can use the floor function to do so.
Another use case for the floor function is in data analysis. When working with data, it is often necessary to group values into discrete bins or categories. The floor function can be used to accomplish this by rounding values down to the nearest integer value and then using those integers to define the categories. For example, if you have a list of floating-point values representing ages and you want to group them into categories such as "under 18", "18-24", "25-34", and so on, you can use the floor function to round the ages down to the nearest integer and then use those integers to define the categories.
What is the difference between floor and ceil in Python
The floor and ceil functions in Python are both used to round a number to a specific precision, but they differ in the direction of rounding.
The floor function rounds a number down to the nearest integer that is less than or equal to the number. For example, if you use floor on the number 4.8, it will return 4. Similarly, if you use floor on the number -4.8, it will return -5.
The ceil function, on the other hand, rounds a number up to the nearest integer that is greater than or equal to the number. For example, if you use ceil on the number 4.8, it will return 5. Similarly, if you use ceil on the number -4.8, it will return -4.
What data types does the floor function work with in Python?
The floor function in Python works with both integer and floating-point numbers. When called with an integer argument, the floor function simply returns the same integer value. When called with a floating-point number, the function rounds down to the nearest integer that is less than or equal to the floating-point number.
It is important to note that the floor function always returns an integer value, even if the input value is a floating-point number. This is because the function is designed to round down to the nearest integer.
The floor function can also work with other numeric data types, such as decimal or fraction objects, as long as they are compatible with the standard numeric operations in Python.
Can the floor function be used for rounding in Python?
Yes, the floor function in Python can be used for rounding, but it is specifically designed to round numbers down to the nearest integer. If you need to round numbers to the nearest integer, then the floor function is a good choice. However, if you need to round numbers to the nearest integer with the standard rounding rules (i.e., rounding up if the decimal component is 0.5 or greater), then you should use the built-in round function instead.
It's important to note that the round function in Python uses a different rounding rule than the floor function. The round function rounds numbers to the nearest integer, and if the decimal component is exactly 0.5, it rounds to the nearest even integer. For example, round(1.5) returns 2, and round(2.5) returns 2.
What happens if you pass a non-numeric value to the floor function in Python
If you pass a non-numeric value to the floor function in Python, you will get a TypeError. The floor function is designed to work with numeric values, specifically integer and floating-point numbers. If you try to pass a non-numeric value, such as a string or a boolean, Python will raise a TypeError indicating that the operation is not supported.
Following is an example of what would happen if you try to pass a string to the floor function:
As you can see, Python raises a TypeError indicating that the '<=' operator is not supported between a string and an integer (which is what the floor function expects).
Python Double Slash (//) Operator and floor
The Python double slash (//) operator performs integer division, which means that it divides two numbers and then rounds the result down to the nearest integer (towards negative infinity). This is similar to the behavior of the floor function in Python.
Consider the following code:
In the above example, use the double slash operator to perform integer division between x and y, which results in a value of 2. This is because 5 / 2 is equal to 2.5, which rounds down to 2 when we use integer division.
The behavior of the double slash operator is similar to the floor function in Python, but there are some important differences. One key difference is that the double slash operator always returns an integer value, whereas the floor function can return a floating-point value. Additionally, the double slash operator always rounds towards negative infinity, whereas the floor function can be used to round towards negative infinity or zero, depending on the input value.
numpy.floor()
The numpy.floor is a function in the NumPy library that behaves similarly to the built-in math.floor function in Python. The numpy.floor function returns the largest integer value that is less than or equal to each element in an input array.
Following is an example of how to use numpy.floor:
In the above example, an input array x containing four floating-point values. Use the numpy.floor function to round down each element in the array to the nearest integer value that is less than or equal to the element. The resulting array y contains the rounded-down values.
One key difference between numpy.floor and the built-in math.floor function in Python is that numpy.floor can be applied to entire arrays, whereas math.floor only operates on individual scalar values. This makes numpy.floor particularly useful for working with large datasets and performing vectorized operations.
Difference between int() and floor() in Python 3
In Python 3, int() and floor() are two different functions that can be used to perform rounding operations, but they have different behavior and are used in different contexts.
The int() function is a built-in Python function that can be used to convert a floating-point value to an integer by rounding towards zero. In other words, int() truncates the decimal part of a floating-point value and returns the integer part.
In the above example, the int() function is used to convert the floating-point value 3.5 to an integer by rounding towards zero. The resulting integer value is 3.
On the other hand, the floor() function is a function from the math module that can be used to round a floating-point value down to the nearest integer that is less than or equal to the value. In other words, floor() always rounds down towards negative
infinity.
In the above example, the math.floor() function is used to round the floating-point value 3.5 down to the nearest integer that is less than or equal to the value. The resulting integer value is also 3.
Conclusion:
The purpose of the floor function in Python is to round a given number down to the nearest integer that is less than or equal to the given number. This function is useful in a variety of situations where integer values are needed, such as financial calculations and data analysis.