How to use a deque in Python
A deque is a double-ended queue that allows you to add or remove elements from both the front and the back of the queue with constant time complexity. The name "deque" is short for "double-ended queue." It is similar to a list in Python, but it provides faster operations for adding or removing elements at both ends of the queue. When elements are added or removed from the front or back of a deque, the other elements in the deque are shifted accordingly.
Doubly-linked list
Deques are implemented in Python using a doubly-linked list, which means that each element in the deque has pointers to both the previous and next elements in the deque. This allows for constant time complexity for operations like adding or removing elements from either end of the deque. Deques can contain elements of any data type, and they can grow or shrink dynamically as elements are added or removed. You can also access elements of a deque by their index, just like a list.
Add elements to the front and back of a deque
You can add elements to the front and back of a deque in Python using the append() and appendleft() methods, respectively.
deque append() method
The append() method adds an element to the back of the deque. It takes one argument, which is the element you want to add to the deque.
In the above example, create an empty deque using the deque() constructor from the collections module. Then add three elements to the back of the deque using the append() method.
deque appendleft() method
The appendleft() method adds an element to the front of the deque. It takes one argument, which is the element you want to add to the deque.
In the above example, create an empty deque using the deque() constructor from the collections module. Then add three elements to the front of the deque using the appendleft() method.
Note that when adding elements to a deque, the order in which you add them determines their position in the deque. The first element you add will be at the front of the deque, and the last element you add will be at the back of the deque.
Remove elements from the front and back of a deque
You can remove elements from the front and back of a deque in Python using the popleft() and pop() methods, respectively.
deque popleft() method
The popleft() method removes the element from the front of the deque and returns it. If the deque is empty, it raises an IndexError.
In the above example, create a deque with three elements using the deque() constructor from the collections module. Then remove the element from the front of the deque using the popleft() method and store it in the variable x.
deque pop() method
The pop() method removes the element from the back of the deque and returns it. If the deque is empty, it raises an IndexError.
In the above example, create a deque with three elements using the deque() constructor from the collections module. Then remove the element from the back of the deque using the pop() method and store it in the variable x.
Note that when removing elements from a deque, the order in which you remove them determines which element is returned. The first element you remove using popleft() will be the first element added to the deque, and the last element you remove using pop() will be the last element added to the deque.
Iterate over a deque object in Python
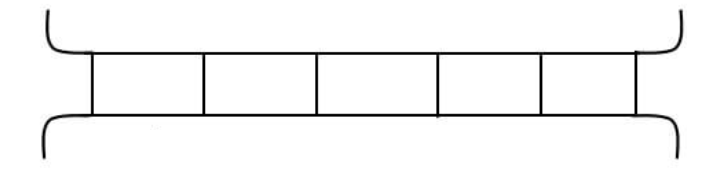
You can iterate over a deque object in Python using a for loop, just like you would with a list.
In the above example, create a deque with three elements using the deque() constructor from the collections module. Then iterate over the elements of the deque using a for loop and print each element.
You can also use other methods provided by the deque object to iterate over its elements, such as __iter__() or iter() methods.
In the above example, create a deque with three elements using the deque() constructor from the collections module. Then use the __iter__() method and iter() method to create an iterator for the deque and use it to iterate over the elements of the deque using a while loop.
Note that the __iter__() method returns an iterator object, and you can use the __next__() method of the iterator object to get the next element of the deque. The iter() function returns an iterator object that you can use with the next() function to get the next element of the deque.
Commonly used functions in deque
In the above example, create an empty deque using the deque() constructor from the collections module. Then add some elements to the deque using the append() method.
To get the length of the deque, use the len() function and pass the deque as an argument. The len() function returns the number of elements in the deque.
To check if the deque is empty, use the boolean operator not and pass the deque as an argument. The not operator returns True if the deque is empty, and False otherwise.
Then remove all elements from the deque using the clear() method and repeat the same steps to get the length and check if the deque is empty.
Common use cases for deques in Python
Deques (short for "double-ended queues") are a versatile data structure in Python that can be used in a variety of contexts. Some common use cases for deques in Python include:
- Implementing a queue: Deques are commonly used to implement queue data structures, where elements are added to the back of the queue and removed from the front. Since deque provides fast operations for both adding and removing elements from both ends of the queue, it can be an efficient choice for implementing a queue.
- Implementing a stack: Similarly, deques can be used to implement stack data structures, where elements are added to the top of the stack and removed from the same location. Since deque provides fast operations for adding and removing elements from both ends, it can be an efficient choice for implementing a stack.
- Implementing sliding windows: Deques can be useful for implementing sliding windows, which are a common pattern in algorithmic problems. In this context, a deque can be used to efficiently keep track of a sliding window of fixed size over a sequence of elements.
- Implementing efficient algorithms: Deques can be useful in implementing certain algorithms that require efficient access to elements at both ends, such as breadth-first search, depth-first search, and Dijkstra's shortest path algorithm.
- Implementing other data structures: Deques can also be used to implement other data structures such as double-ended priority queues and double-ended heaps.
Advantages of using a deque over a list in Python
There are several advantages of using a deque over a list in Python:
- Faster Appends and Pops: Deque is implemented using a doubly linked list, which allows for constant time operations for adding or removing elements from both ends of the deque. On the other hand, list operations for adding or removing elements from the beginning of a list can take up to linear time complexity.
- Memory Efficiency: Since a deque is implemented as a doubly linked list, it does not need to reallocate memory when it grows or shrinks, which can make it more memory efficient than a list.
- Thread-safety: Deques provide a thread-safe implementation, which means that multiple threads can safely access the same deque object without any data corruption or inconsistency issues.
- Better performance for specific use cases: Deques are designed to handle specific use cases such as implementing queues and stacks, and can provide better performance than a list in those cases. For example, if you're implementing a queue data structure, a deque can provide O(1) time complexity for both enqueue and dequeue operations, while a list would provide O(n) time complexity for dequeuing elements from the beginning of the list.
- Rotate Operation: A deque provides a rotate() method that can be used to efficiently rotate the deque in either direction. This operation can be useful in implementing certain algorithms and data structures.
Conclusion:
A deque in Python is a data structure that allows you to add or remove elements from both ends of the queue with constant time complexity. It is implemented using a doubly-linked list and is useful for situations where you need to access both ends of a queue frequently and efficiently.