How to Use Do-While loop in Python
A do-while loop is a type of loop that executes a block of code at least once, and then continues to execute the block of code as long as a certain condition is true. The difference between a do-while loop and a regular while loop is that in a do-while loop, the code block is executed before the condition is checked, while in a while loop, the condition is checked before the code block is executed.
Syntax:Here, the code block is executed at least once, and then the condition is checked. If the condition is true, the loop continues to execute the code block; otherwise, the loop is exited.
The do-while loop is useful in situations where you want to ensure that a certain block of code is executed at least once, regardless of whether the condition is initially true or false. For example, if you want to prompt a user to enter a password and continue prompting them until they enter the correct password, a do-while loop would be useful to ensure that the prompt is displayed at least once.
Python do-while loop
In Python, there is no built-in do-while loop, but you can achieve the same functionality using a while loop with a break statement. The while loop is similar to the do-while loop in that the code block is executed as long as the condition is true, but the difference is that the condition is checked before the code block is executed. Therefore, to emulate a do-while loop in Python, you would need to structure your code so that the code block is executed at least once, and then use a while loop with a break statement to exit the loop when the condition is no longer true.
Using while loop
Following is an example of how you can implement a do-while loop in Python:
In the above example, use a while loop with an infinite condition (True) and a break statement to exit the loop when the condition is no longer true.
Let's see an example of using a do-while loop to calculate the sum of integers from 1 to n:
In the above example, start with n = 5 and initialize the sum variable to zero. Inside the loop, add n to sum and decrement n by 1. The loop continues until n is equal to zero, at which point the break statement is executed, and the loop is exited. Finally, print the value of sum.
As you can see, the loop executed five times (once for each integer from 1 to 5) and calculated the correct sum.
Why Python does not have a "do-while" loop?
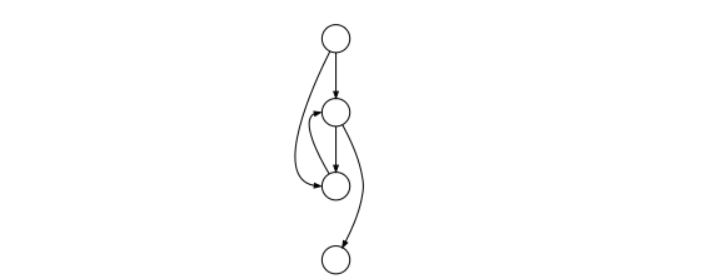
Python does not have a built-in do-while loop because it is considered less necessary in Python than in other programming languages.
Python's while loop can be used to achieve the same functionality as a do-while loop by simply executing the code block before checking the condition, which ensures that the code block is executed at least once. The while loop checks the condition at the beginning of each iteration, which allows for the same level of control as a do-while loop.
Conclusion
Python is designed to be a high-level, easy-to-read language that emphasizes simplicity and readability over complex syntax. The lack of a do-while loop in Python simplifies the language's syntax and reduces the number of control structures that a programmer needs to learn, making it easier to read and write Python code.