Implementing an Interface in Python
In Python programming, an interface is a blueprint or a contract that defines a set of methods, properties, and behaviors that a class must implement. It is a way to establish a common communication protocol between two components of a program.
Interface
An interface provides a way to enforce a specific structure for the class that implements it. It defines the method signatures (the names, parameters, and return types of the methods) that a class must implement to conform to the interface. It does not contain the actual implementation of the methods, only the method signatures.
When a class implements an interface, it guarantees that it will provide the methods and behaviors specified by the interface. This allows other classes to use the interface without needing to know about the implementation details of the implementing class. This separation of concerns allows for greater modularity and flexibility in a program's design.
Python interface
Python does not have a specific keyword or syntax for defining an interface like some other programming languages. Instead, a Python interface is often created using an abstract base class. An abstract base class is a class that cannot be instantiated and is used to define a common interface for its subclasses. The abstract base class defines the required methods and behaviors that its subclasses must implement to conform to the interface.
Benefits of using a Python interface
There are several benefits to using a Python interface:
- Modularity: Interfaces promote modularity in a program's design by separating concerns. By defining a common interface for a set of related classes, we can easily swap out one implementation with another without affecting the rest of the program.
- Abstraction: An interface provides an abstraction layer that allows us to write code that is not tied to any particular implementation. This abstraction helps to reduce coupling between different parts of the program, which makes it easier to modify and maintain the code over time.
- Polymorphism: Interfaces enable polymorphism, which allows different objects to be used interchangeably in a program. By defining a common interface, we can treat different objects as if they were the same type, which makes it easier to write generic code that works with a wide range of objects.
- Code reuse: Interfaces allow us to define a set of behaviors that can be reused across multiple classes. This code reuse helps to reduce duplication and makes the code easier to maintain.
- Testing: Interfaces provide a way to test classes in isolation. By defining a common interface, we can write test cases that work with any class that implements the interface. This makes it easier to test the behavior of individual classes without needing to test the entire program at once.
- Collaboration: Interfaces enable collaboration between different developers by providing a common language that they can use to communicate about the behavior of the program. By defining a common interface, developers can agree on a set of behaviors that their classes must implement, which helps to reduce confusion and ensure that the program behaves correctly.
Informal Interfaces in Python
An informal interface is a convention that specifies a set of methods that a class should implement, but without using a formal interface or abstract base class. Informal interfaces are also sometimes referred to as "duck typing" because if it looks like a duck and quacks like a duck, it's a duck.
Informal interfaces are often used in Python because the language does not require explicit implementation of an interface. Instead, if a class has the necessary methods to behave like an interface, it can be used as one. This approach makes Python flexible and easy to use, but it also requires a degree of trust and responsibility on the part of the programmer to ensure that their code conforms to the informal interface.
Following is an example of an informal interface in Python:
In the above example, defined a class called Calculator that has four methods: add, subtract, multiply, and divide. These methods define the basic functionality of a calculator, but there is no formal interface or abstract base class that specifies these methods.
You can use this class as an informal interface by creating a subclass that implements all of the necessary methods:
In the above example, created a subclass called ScientificCalculator that extends the Calculator class and adds three new methods: sin, cos, and tan. By extending the Calculator class, implicitly stating that the ScientificCalculator class conforms to the informal interface specified by the Calculator class.
You can then use instances of the ScientificCalculator class anywhere that a Calculator object is expected, because it implements all of the necessary methods. This demonstrates the flexibility and power of informal interfaces in Python.
Formal Interfaces in Python
Formal interfaces can be defined using either abstract base classes (ABCs) or protocols. Both approaches allow us to define a formal contract that specifies a set of methods and properties that a class must implement in order to conform to the interface. Using formal interfaces can help to enforce code contracts and make code more predictable and easier to maintain.
Using an Abstract Base Class (ABC)
Following is an example of a formal interface defined using an abstract base class in Python:
In the above example, defined an abstract base class called Animal that specifies two abstract methods: speak and move. Any class that wants to conform to this interface must implement these methods. If a class does not implement these methods, attempting to create an instance of that class will result in an error.
Following is an example of a class that conforms to the Animal interface:
In the above example, defined a class called Dog that extends the Animal abstract base class and implements the speak and move methods. By extending the Animal class, you are explicitly stating that the Dog class conforms to the Animal interface.
Using a Protocol
Following is an example of a formal interface defined using a protocol in Python:
In the above example, defined a protocol called Serializable that specifies two methods: serialize and deserialize. Any class that wants to conform to this protocol must implement these methods. If a class does not implement these methods, attempting to create an instance of that class will result in an error.
Following is an example of a class that conforms to the Serializable protocol:
In the above example, defined a class called Employee that implements the serialize and deserialize methods defined in the Serializable protocol. By doing so, explicitly stating that the Employee class conforms to the Serializable protocol.
Popular Python interfaces
There are many popular Python interfaces that are widely used in various applications and frameworks. Here are some examples of popular Python interfaces:
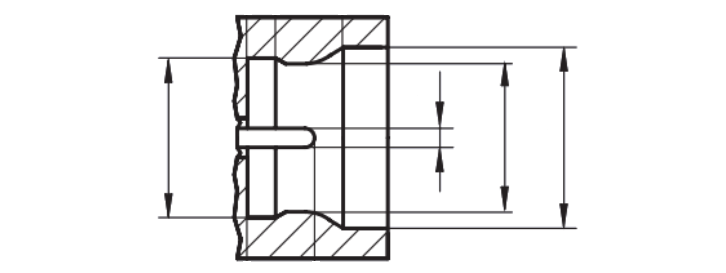
- Abstract Base Classes (ABCs): ABCs are a powerful way to define interfaces in Python. They allow you to specify a set of methods that a class must implement in order to conform to the interface. Some of the most commonly used ABCs in Python include collections.abc.Iterable, collections.abc.Mapping, and collections.abc.Callable.
- Protocols: Protocols are a newer way to define interfaces in Python 3.8 and later. They allow you to specify a set of methods and/or attributes that a class must implement in order to conform to the interface. Some commonly used protocols in Python include typing.Protocol and typing.TypedDict.
- Django ORM: The Django Object-Relational Mapper (ORM) provides a powerful interface for interacting with databases in Python. It allows you to define models that map to database tables and provides methods for querying and manipulating data.
- PyTorch: PyTorch is a popular machine learning library that provides a Python interface for working with tensors and building neural networks. It provides a wide range of functions and classes for manipulating tensors and building models.
- Flask: Flask is a lightweight web framework for Python that provides a simple and flexible interface for building web applications. It provides methods for defining routes, handling requests and responses, and rendering templates.
Difference between a Python interface and a Python library
A Python interface and a Python library are two different concepts in Python programming.
Python Interface:
A Python interface is a set of methods and properties that a class must implement in order to conform to the interface. An interface is used to define a contract for how classes should behave and interact with each other. Interfaces are not directly executable code, but rather a set of rules that must be followed.
Python Library:
A Python library is a collection of reusable code modules that can be imported and used in Python programs. Libraries are designed to provide specific functionality or solve specific problems, such as working with databases, parsing data formats, or building graphical user interfaces. Libraries can be executed directly as part of a program and can be called using specific function calls.
Conclusion
Interfaces are not explicitly defined like in some other programming languages. Instead, interfaces are implemented using abstract base classes (ABCs) or by defining classes with specific methods that need to be overridden by the implementing class. By adhering to the required method signatures and behaviors, a class can effectively implement the desired interface in Python.