How to use Reduce() Function in Python
The Python reduce() function is a built-in function in the functools module that is used for iterative operations. It takes two arguments, a function and an iterable, and applies the function to the elements of the iterable in a cumulative way.
A simple example of Python reduce()
Following is a simple example of how to use the reduce() function to find the sum of elements in a list:
In the above example, import the reduce() function from the functools module and define a list of numbers. Then use the reduce() function to find the sum of the numbers in the list by passing a lambda function that takes two arguments (x and y) and returns their sum. The reduce() function applies this lambda function to the first two elements of the list (1 and 2) to get 3, then applies the function to 3 and the next element (3) to get 6, and so on until it has applied the function to all the elements in the list, resulting in the final output value of 15.
What is the Python reduce function and what does it do
The reduce() function starts by applying the given function to the first two elements of the iterable. It then takes the result of this operation and applies the function to it and the next element in the iterable. This process continues until there are no more elements in the iterable, resulting in a single output value.
In other words, the reduce() function "reduces" the iterable to a single value by combining the elements in a cumulative way using the given function. The function can be any valid Python function, including a lambda function or a user-defined function.
One important thing to note about the reduce() function is that it requires an iterable with at least two elements. If the iterable has only one element, the function will return that element as the output value. If the iterable is empty, an error will be raised.
The reduce() function is a powerful tool that can be used for a variety of tasks, including finding the sum or product of the elements in an iterable, concatenating strings, and finding the maximum or minimum value in a list.
Multiplying Numeric Values
Following is an example of how to use reduce() to multiply the numeric values in a list:
In the above example, define a list of numbers and use the reduce() function to find the product of the numbers in the list by passing a lambda function that takes two arguments (x and y) and returns their product. The reduce() function applies this lambda function to the first two elements of the list (2 and 3) to get 6, then applies the function to 6 and the next element (4) to get 24, and so on until it has applied the function to all the elements in the list, resulting in the final output value of 120.
Finding the Minimum and Maximum Value
Following are examples of how to use reduce() to find the minimum and maximum values in a list:
In the above examples, define a list of numbers and use reduce() with lambda functions to find the minimum and maximum values in the list. For the minimum value, pass a lambda function that takes two arguments (x and y) and returns the smaller of the two values using a ternary operator. The reduce() function applies this lambda function to the first two elements of the list (3 and 1) to get 1, then applies the function to 1 and the next element (4) to get 1, and so on until it has applied the function to all the elements in the list, resulting in the final output value of 1. For the maximum value, pass a similar lambda function that returns the larger of the two values using a ternary operator. The reduce() function applies this lambda function to the first two elements of the list (3 and 1) to get 3, then applies the function to 3 and the next element (4) to get 4, and so on until it has applied the function to all the elements in the list, resulting in the final output value of 9.
Checking if All Values Are True
Following is an example of how to use reduce() to check if all values in a list are true:
In the above example, define a list of boolean values and use reduce() with a lambda function to check if all values in the list are true. The lambda function takes two arguments (x and y) and uses the logical AND operator (and) to check if both values are true. The reduce() function applies this lambda function to the first two elements of the list (True and True) to get True, then applies the function to True and the next element (False) to get False, and so on until it has applied the function to all the elements in the list, resulting in the final output value of False.
Checking if Any Value Is True
Following is an example of how to use reduce() to check if any value in a list is true:
In the above example, define a list of boolean values and use reduce() with a lambda function to check if any value in the list is true. The lambda function takes two arguments (x and y) and uses the logical OR operator (or) to check if either value is true. The reduce() function applies this lambda function to the first two elements of the list (False and False) to get False, then applies the function to False and the next element (True) to get True, and so on until it has applied the function to all the elements in the list, resulting in the final output value of True.
Difference between the reduce function and the map function
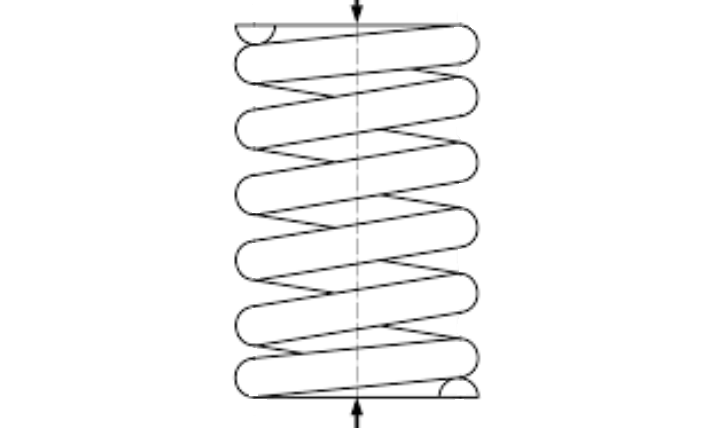
The reduce() function and the map() function are both higher-order functions in Python that operate on sequences (e.g., lists, tuples). However, they serve different purposes and have different return types.
map() function
The map() function applies a function to every element of a sequence and returns a new sequence with the results. The length of the new sequence is the same as the original sequence. The syntax for the map() function is as follows:
Here, function is the function to apply to each element in sequence. The map() function applies function to each element in sequence and returns a new sequence with the results.
For example, if you want to apply the square function to every element in a list, you can use the map() function as follows:
In the baove case, the map() function applies the lambda function to every element in the numbers list and returns a new sequence of the resulting squares.
reduce() function
The reduce() function, on the other hand, applies a function to a sequence of values and returns a single value. The reduce() function takes the first two elements of the sequence, applies the function to them, and then applies the function to the result and the next element in the sequence, and so on, until it has processed all the elements in the sequence. The syntax for the reduce() function is as follows:
Here, function is the function to apply to the sequence of values.
For example, if you want to find the product of a list of numbers using reduce(), you can do the following:
In the above case, the reduce() function applies the lambda function to the first two elements of the numbers list (1 and 2) to get 2, then applies the lambda function to 2 and the next element (3) to get 6, and so on, until it has applied the lambda function to all the elements in the list, resulting in the final output value of 120.
Conclusion:
The map() function returns a sequence with the same length as the original sequence, where each element is the result of applying a function to the corresponding element in the original sequence. The reduce() function returns a single value that is the result of applying a function to a sequence of values.