Replacements for switch statement in Python
Switch case statements are useful in programming when you need to evaluate a single expression and perform different actions based on its value. It provides a clear and concise way to handle multiple cases and can make code more readable and maintainable.
Python Match-Case Statement
Starting from Python 3.10, the match statement has been introduced as a replacement for switch statements. It allows you to perform pattern matching on a given value and execute the corresponding block of code based on the matched pattern. Following is an example of how to use the new match statement in Python 3.10 to implement the calculate() function:
In the above example, use the new match statement to perform a pattern match on the operator argument. Define five patterns: one for each of the four arithmetic operators (+, -, *, and /) and one for the default case, represented by the wildcard pattern _. For each of the four arithmetic operator patterns, provide the corresponding expression to evaluate when the pattern is matched. For the default case, provide the error message expression "Invalid operator".
Using the match statement can make the code more concise and readable, especially when there are many cases to consider. The match statement can also provide more flexibility and power than other techniques, such as if and else statements or dictionary mapping, because it can handle more complex patterns and expressions. However, it requires Python 3.10 or later, so it may not be compatible with older versions of Python.
What is the replacement of Switch Case in Python?
Upto Python 3.10, Python does not have a built-in switch statement like some other programming languages such as C/C++, Java, or JavaScript. However, you can emulate the behavior of a switch statement using a combination of if, elif, and else statements or by using a dictionary mapping etc...
Switch Case implement in Python using if-else
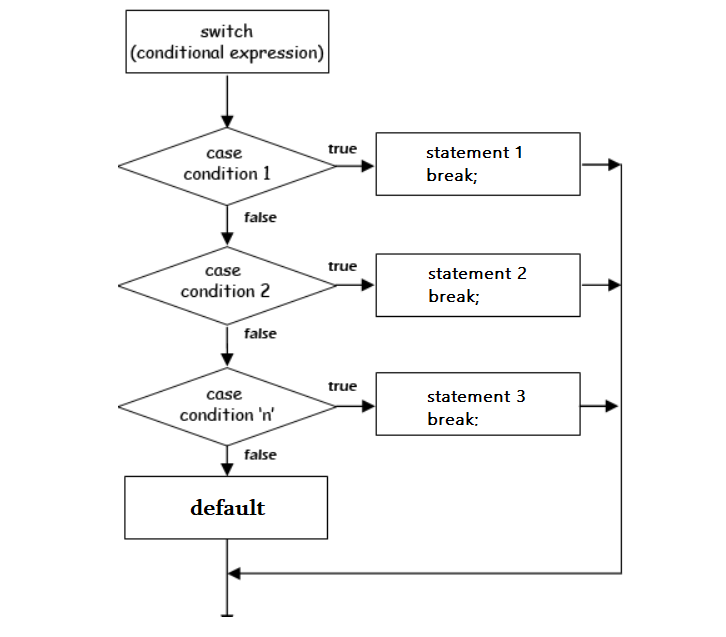
You can emulate the behavior of a switch statement using a combination of if, elif, and else statements. Following is an example of how to implement a switch statement using if and else:
In the above example, the calculate() function takes three parameters: an operator (+, -, *, or /), and two operands (operand1 and operand2). The function uses if and elif statements to check which operator was passed in and performs the corresponding arithmetic operation. If the operator is not one of the four allowed values, the function returns an error message.
Switch Case implement in Python using Dictionary Mapping
You can also emulate the behavior of a switch statement using a dictionary mapping. Following is an example of how to implement a switch statement using dictionary mapping:
In the above example, the calculate() function takes three parameters: an operator (+, -, *, or /), and two operands (operand1 and operand2). The function uses a dictionary mapping to associate each operator with its corresponding arithmetic operation. The get() method is used to retrieve the corresponding operation from the dictionary. If the operator is not one of the four allowed values, the function returns an error message.
Switch Case implement in Using Python Functions
You can also emulate the behavior of a switch statement using functions. Following is an example of how to implement a switch statement using functions:
In thje above example, define four functions that perform each of the arithmetic operations: add(), subtract(), multiply(), and divide(). Then, define the calculate() function that takes three parameters: an operator (+, -, *, or /), and two operands (operand1 and operand2). The calculate() function uses a dictionary mapping to associate each operator with its corresponding arithmetic function. The get() method is used to retrieve the corresponding function from the dictionary. If the operator is not one of the four allowed values, the function returns an error message.
Switch Case implementation using lambda functions
You can also emulate the behavior of a switch statement using lambda functions.
In the above example, define a dictionary of lambda functions that perform each of the arithmetic operations. Then, define the calculate() function that takes three parameters: an operator (+, -, *, or /), and two operands (operand1 and operand2). The calculate() function uses a dictionary mapping to associate each operator with its corresponding lambda function. The get() method is used to retrieve the corresponding lambda function from the dictionary. If the operator is not one of the four allowed values, the function returns a lambda function that returns an error message.
Conclusion
The traditional switch statement is not available, but there are several alternative approaches to achieve similar functionality. One common replacement is using a dictionary mapping keys to functions, where the keys correspond to the different cases, allowing for efficient and concise branching logic. Another approach involves using if-elif-else constructs to simulate the behavior of a switch statement, providing a more flexible way to handle multiple cases based on conditions.