Python Dictionary Comprehension
In Python, a comprehension stands as an elegantly succinct technique to construct complex data structures via the utilization of iterators. Specifically, a dictionary comprehension represents a streamlined approach to fashioning a fresh dictionary in Python. This construct mirrors the concept of list comprehension, yet diverges by crafting a dictionary instead of a list, thus contributing to the code's conciseness and readability while adeptly managing data transformation.
Syntax:For example, to create a dictionary that maps integers to their squares, you can use the following dictionary comprehension:
This creates a dictionary with keys from 0 to 5 and values as their square.
Without Dictionary Comprehension
Presented below is an alternative implementation that accomplishes the intended outcome without resorting to the utilization of Dictionary Comprehension. However, this approach necessitates the inclusion of a more extensive code segment. The adoption of Dictionary Comprehension not only obviates the need for an extended code base but also contributes to sustaining the pristine clarity and organization of your code. This demonstrates the inherent advantage of employing Dictionary Comprehension as it efficiently optimizes code length while preserving its overall legibility.
Dictionary Comprehension | Example
A simple dictionary comprehension that uses string as an iterable.
Dictionary Comprehension from two iterables
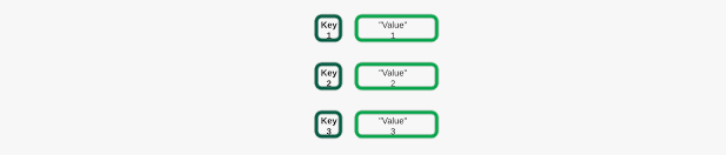
Here there are two lists named keys and value and iterating over them with the help of zip() function.
Dictionary comprehension | Enumerate
By using enumerate() method, you can create a dictionary from the list with list index number as key and list element as value.
Dictionary comprehension | if clause
Dictionary comprehension can also include an optional if clause, like this:
This creates a dictionary with only even keys and their square values.
Dictionary comprehension | Extracting a subset
If you want to select some items from Dictionary, you can use Dictionary comprehension to extract particular keys and values from a dictionary.
Nested Dictionary Comprehension
Here is an example for nested Dictionary Comprehension.
Dictionary Comprehension | Advantages
- They are easy to read and understand, as the syntax is similar to list comprehensions and follows a clear, logical structure.
- They can be used to perform complex operations on dictionaries, such as filtering, mapping, and aggregating items, in a single line of code.
- They can be more memory-efficient than using for loops, as the items are generated on the fly, rather than being stored in a separate list or variable.
- They can be faster than using for loops, as the operations are applied directly to the items, rather than iterating over them multiple times.
Dictionary Comprehension | Disadvntages
- They may be less readable or more difficult to understand for people who are not familiar with the syntax.
- They can be less flexible than using for loops, as they are limited to a single expression and do not allow for multiple statements or control flow.
- They may be less efficient for very large dictionaries or when dealing with complex operations that cannot be expressed in a single expression.
- They can be less explicit and harder to debug when the code fails.
- They can be less explicit when you are trying to filter the dictionary based on multiple conditions.
Conclusion
Python Dictionary Comprehension is a concise and efficient method to create dictionaries in Python by specifying key-value pairs within a compact syntax. It offers a streamlined alternative to traditional methods, allowing developers to create dictionaries with less code and improved readability. By using dictionary comprehension, you can construct dictionaries with ease while maintaining code clarity and conciseness.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python