Python Modulo Operator (%)
In Python, the modulo operator (%) is used to find the remainder when one number is divided by another. It calculates the remainder after performing integer division between two operands.
Basic Usage
In this example, the remainder when 10 is divided by 3 is 1.
Checking for Even or Odd
Here, we use the modulo operator to check if the number is even or odd. If the remainder of the number divided by 2 is 0, it's even; otherwise, it's odd.
Finding Leap Years
In this example, we determine if a year is a leap year using the modulo operator. A leap year is either divisible by 4 but not by 100, or it is divisible by 400.
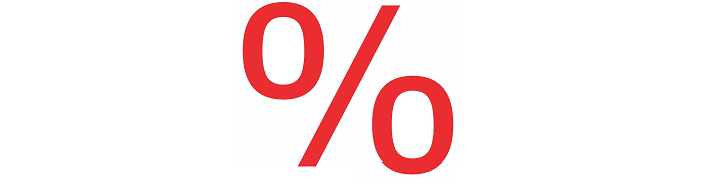
Here are some other examples of how the Python modulo operator can be used:
- To check if a number is a multiple of another number.
- To calculate the remainder of a division operation.
- To calculate the number of times a number divides evenly into another number.
- To generate a sequence of numbers.
Modulo Operation in Mathematics
The modulo operation, often referred to as "clock arithmetic," yields a result that always falls within the range of 0 to (divisor-1). This arithmetic operation finds wide application in diverse fields of mathematics, encompassing number theory, cryptography, and computer science. Its versatility stems from its ability to determine remainders, making it a valuable tool for various algorithms, calculations, and problem-solving techniques.
The modulo operation provides a fundamental mechanism for handling periodic or cyclic phenomena, as it effectively wraps around a cyclical sequence, such as a clock. By confining the output to a limited range, it simplifies calculations involving repetitions, rotations, and periodicity. This property proves particularly valuable in applications related to encryption, hashing, data structures, and data partitioning, enhancing the efficiency and security of algorithms in computer science. In number theory, the modulo operation plays a key role in various concepts, including congruences, modular arithmetic, and primality testing, offering profound insights into the properties of numbers and mathematical relationships.
Conclusion
The modulo operator is commonly used in various scenarios, such as controlling loops, validating divisibility, and handling circular data structures. It's a powerful tool for performing arithmetic operations that involve remainders in Python.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python