Python print() to File
In Python, you can use the print() function not only to display output on the screen but also to write content to a file. This is achieved by specifying the file parameter when calling the print() function. Let's explore how to write to a file using the print() function with examples:
Writing to a Text File
In this example, the with statement is used to open a file named "output.txt" in write mode. The print() function is then utilized to write two lines of text to the file. The file parameter is set to the opened file object, allowing the print() function to write directly to the file.
Appending to a File
In this example, the file is opened in append mode using the "a" parameter. This allows the print() function to append content to the existing file without overwriting its contents.
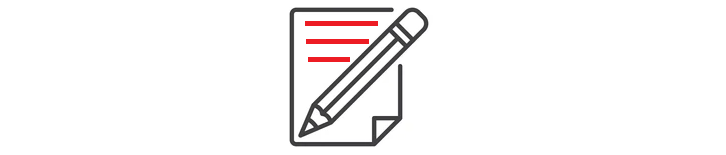
By using the print() function to write to files, you can easily format the output and control line breaks. You can also include variables and expressions within the print() function to write dynamic content to the file.
Python print()
The Python print() function serves as a essential tool for generating textual or data output within the console environment. This versatile function not only facilitates the display of variables, strings, and numerical values, but also extends its utility to encompass various data types. Importantly, the print() function offers enhanced customization through the incorporation of optional parameters, such as end, sep, file, and flush, enabling precise control over the function's behavior during execution.
As a fundamental component of Python's standard library, the print() function contributes significantly to the readability and functionality of programs. It empowers developers to communicate program results, intermediate calculations, and informative messages in a manner that aligns with their intended objectives.
Conclusion
It's important to note that while using print() to write to files is suitable for basic text-based output, for more complex file writing tasks or when dealing with non-text files, the write() method and other file-related functions provided by the Python io module may be more appropriate.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python