Convert integer to string in Python
The conversion of an integer to a string in Python bears significance across a multitude of contexts, proving particularly valuable when endeavoring to amalgamate integers with strings within print statements or when supplying integers as parameters to functions that necessitate string inputs. This transformation, effectuated by converting an integer to a string, guarantees the precise interpretation of values throughout your codebase, thereby fortifying the integrity of your program's functionality and ensuring seamless compatibility among varying data types.
Using str() function
In Python, you can convert an integer to a string using the str function.
As you can see, calling str(x) will return a string representation of the integer x. The type of the resulting string is str.
Using .format() function
The format() method presents an alternative avenue for converting an integer to a string within the Python context. Its utility lies in its capacity to incorporate values into a string, proving particularly advantageous when the need arises to seamlessly integrate values into a string while adhering to precise formatting requirements. This mechanism becomes notably advantageous in scenarios where controlled and structured embedding of data is imperative for achieving the desired representation.
In the above example, the {} is a placeholder for a value, and the format() method is used to insert the value of x into the placeholder. This will return a string representation of the integer x, and the type of the resulting string is str.
Using f-string
An f-string in Python represents a specific genre of string literal that enables the seamless integration of expressions within the string content, employing the usage of curly braces {} as designated receptacles for these dynamic values. Here's an example of how you can use an f-string to convert an integer to a string:
In the above example, the expression x inside the curly braces {} is evaluated, and its value is inserted into the string. The resulting string is a string representation of the integer x, and the type of the resulting string is str.
Using the "%s" integer
The % operator serves as an additional tool for string formatting. When employed in conjunction with a string, it functions as a placeholder for values, facilitating the conversion of an integer to a string within the string formatting context. Here's an example:
In the above example, the string "%s" acts as a placeholder for a value, and the % operator is used to insert the value of x into the placeholder. This will return a string representation of the integer x, and the type of the resulting string is str.
It is important to note that the %s placeholder is used to format a value as a string, so it can be used to format values of any type, including integers.
Using Integer.__str__()
You can also convert an integer to a string in Python by using the __str__ method, which is a built-in method for converting objects to strings. Here's an example:
In the above example, the __str__ method is used to convert the integer x to a string, and the result is stored in the variable x_str. The type of x_str is str.
The str function and the direct invocation of the str method are functionally interchangeable. Both mechanisms yield a string representation of an integer, thereby enabling the conversion of an integer to a string within the Python context. Consequently, either of these methods can be employed to achieve the desired outcome of converting an integer to a string.
What occurs when an integer is converted to a string in Python?
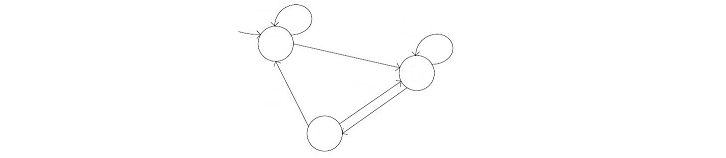
When effecting the conversion of an integer to a string in Python, you initiate the creation of a distinct string object that encapsulates the integer's representation in string format. This string representation fundamentally translates the integer's numerical value into a sequential arrangement of characters.
For instance, converting the integer 23 to a string yields the string "23". The resultant string inherently belongs to the 'str' data type category, mirroring the transformation from numerical data to textual representation.
Conclusion
Converting an integer to a string entails generating a new string object that encapsulates the integer's representation as a sequence of characters. This transformation is essential for scenarios where integers need to be treated as strings for various operations, like concatenation or formatting. The process involves using various methods such as the str() function, f-strings, or the % operator, ultimately producing a string equivalent of the original integer value.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- Random Float numbers in Python