How to delete the last element in a list in Python
In Python, you can delete the last element in a list using either the "del" statement or the "pop()" method. Let's explore each method with examples:
Using the "del" statement
The "del" statement allows you to remove an element from a list by specifying its index. To delete the last element, you can use the index "-1," which represents the last position in the list.
Using the "pop()" method
The "pop()" method is a list method that removes and returns the element at a specified index. If no index is provided, it automatically removes the last element from the list.
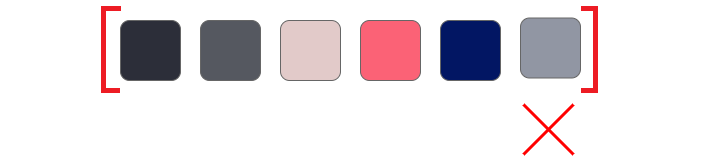
Using the slice() operator
Here is an explanation of how each of these methods works:
- The pop() method removes the element at the specified index from the list. If no index is specified, the pop() method removes the last element in the list.
- The del statement deletes the element at the specified index from the list. If no index is specified, the del statement deletes the last element in the list.
- The slice() operator returns a new list that is a copy of the original list, but with the specified elements removed. In this case, the slice() operator is used to return a new list that is a copy of the original list, but without the last element.
Conclusion
To delete the last item in a list in Python, you can use the "del" statement with the index "-1" or the "pop()" method without any arguments. Both methods effectively remove the last element from the list, adjusting its length accordingly. If you don't need the removed element, using "pop()" without arguments is more convenient, while "del" is a concise option when the deleted value is not required.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python