Convert List to String in Python
There are several ways to convert a list to a string in Python. Here are a few examples:
Using the join() method
The join() method takes a string as an argument and joins the elements of the list together with the string. For example, the following code converts the list ["hello", "world"] to the string "hello world":
Using the str() function
The str() function takes an iterable as an argument and returns a string representation of the iterable. For example, the following code converts the list ["hello", "world"] to the string "hello world":
Using the format() method
The format() method takes a format string and an iterable as arguments and returns a string representation of the iterable. For example, the following code converts the list ["hello", "world"] to the string "hello world":
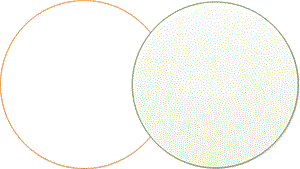
Here is an explanation of how each of these methods works:
- The join() method takes a string as an argument and joins the elements of the list together with the string. The string is repeated for each element in the list. For example, if the string is " ", then the join() method will join the elements of the list together with a space between each element.
- The str() function takes an iterable as an argument and returns a string representation of the iterable. The string representation of the iterable is the result of calling the __str__() method on each element in the iterable.
- The format() method takes a format string and an iterable as arguments and returns a string representation of the iterable. The format string is a template that is used to format the elements of the iterable. For example, the format string "%s" can be used to format the elements of the iterable as strings.
It's important to note that the join() method is efficient for merging elements of a list into a string, especially when the list contains string elements. However, for more complex scenarios where additional formatting is required, using string formatting might be more suitable.
Conclusion
Above methods provide a convenient way to create a coherent string representation from a list of elements, a common operation when generating formatted output or constructing data representations.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to convert int to string in Python
- Random Float numbers in Python