Remove first n characters from a string
In Python, the task of removing the initial 'n' characters from a string can be effectively achieved through slicing. The slice notation [n:] allows the elegant extraction of all characters in the string, commencing from the nth index position. This streamlined approach in Python empowers developers to effortlessly manipulate strings with precision and efficiency.
Using Python slicing
Here is an example of how you can use Python slicing to remove the first 2 characters from a string:
Using negative indexing
You can also use negative indexing to remove characters from the end of the string.
Using string.lstrip() method
You can also use string.lstrip() method to remove specific characters from the left side of the string.
Note that this method only removes characters from the left side of the string, if you need to remove from both side use string.strip() method.
Using string.replace() method
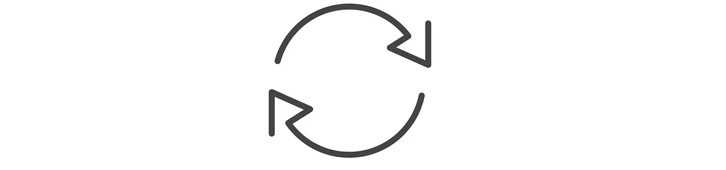
You can also use the string.replace() method to remove specific characters from a string.
These are some examples of how you can remove the first n characters from a string in Python. Depending on your use case, one of these methods may be more appropriate than the others.
Conclusion
To remove the first 'n' characters from a string in Python, you can utilize slicing with the notation [n:]. This method efficiently extracts the desired substring starting from the nth index, offering a simple and effective solution for string manipulation.
- Print the following pattern using python
- Python Program to Check Leap Year
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python