Python enumerate() Function
In Python, the enumerate() function is a built-in function that allows you to iterate over a sequence (such as a list, tuple, or string) while keeping track of the index or position of each element. The enumerate() function returns an iterable object that produces tuples containing both the index and the corresponding element in the sequence.
Enumerate a List
In this example, the enumerate() function is used to loop through the fruits list. It assigns the index of each element to the variable index, and the element itself to the variable fruit. The loop then prints both the index and the corresponding fruit.
Enumerate with a Custom Start Index
In this example, the enumerate() function starts the index from 1 instead of the default 0. This is achieved by passing the start=1 argument to the function.
Enumerate a String
In this example, the enumerate() function is used to iterate through each character in the message string. It assigns the index of each character to the variable index, and the character itself to the variable char.
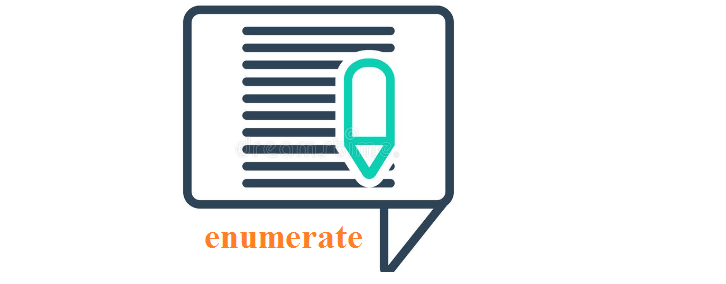
Python Enumerate Vs Python For Loops
The enumerate() function in Python provides the index of the current element along with its corresponding value. In contrast, a for loop enables sequential iteration over the elements in a sequence, allowing you to perform a specific action for each element. While both approaches facilitate iteration over a sequence, the enumerate() function grants access to the index of the current element, which can be particularly useful in certain situations, providing valuable positional information during the iteration process.
Conclusion
The enumerate() function is a handy tool whenever you need to access both the index and the value of elements while iterating through a sequence. It simplifies code and makes it more readable, especially in scenarios where you require the position information along with the element.
- Print the following pattern using python
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python