Print the following pattern using python
Here's one way to print the pattern using a nested loop:
Explanation:
- The outer loop for i in range(1,6) will iterate through the numbers 1 to 5.
- The inner loop for j in range(i) will iterate through the numbers 0 to i-1.
- The print(i, end=" ") statement inside the inner loop will print the current value of i and the end=" " will add a space after the number instead of a newline.
- The print() statement outside the inner loop will add a newline after each row of numbers.
You can also use the * operator to repeat the number i times in each row.
Using Python String join() Method
You can also use join() method of a string to join the repeated characters.
Python print()
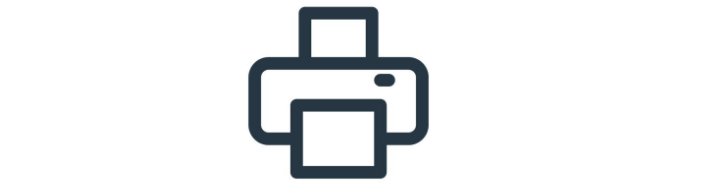
In Python, the print() function serves as a fundamental tool for displaying text or data to the console. This function receives the desired text or data as an argument, enabling the programmer to effortlessly produce output. For instance, by calling print("Hello, world!"), the console would exhibit the message "Hello, world!".
Furthermore, print() can be utilized to exhibit the value of a variable. For instance, if we have the variable x assigned as x = 5, invoking print(x) would produce the value of x, which is 5, in the console.
- Python Program to Check Leap Year
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python