Python Program to Check Leap Year
A leap year in the Gregorian calendar is a year that satisfies the condition of being divisible by 4, excluding those end-of-century years that are not divisible by 400. This mechanism is employed to compensate for the imprecisions in the Earth's orbit, ensuring that the calendar remains synchronized with the astronomical seasons over time.
The concept of a leap year, devised to maintain temporal accuracy, plays a crucial role in aligning the calendar year with the solar year. By establishing the rules for divisibility by 4 and 400 for standard and end-of-century years, respectively, this system effectively accommodates the additional time it takes for the Earth to complete one orbit around the sun, thus preserving the temporal harmony essential for various societal and astronomical applications.
Here is a Python program that checks if a given year is a leap year or not:
Here, the program checks if the year is divisible by 4 and either not divisible by 100 or divisible by 400. If the year satisfies this condition it is considered as leap year otherwise not.
You can also use a function to check for leap year like this:
In this example, the input year is passed as an argument to the function is_leap_year(), which returns True if the year is a leap year and False otherwise. The result is then used to print the appropriate message.
Leap Year
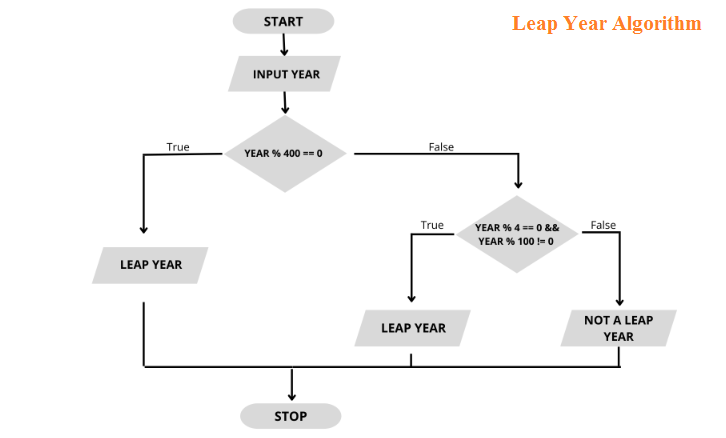
A leap year is a year that satisfies the criterion of being divisible by 4, except for end-of-century years, which must also be divisible by 400. This arrangement is implemented to maintain synchronization between the calendar and the solar year, which spans approximately 365.25 days. By including leap years in the Gregorian calendar, which is widely adopted worldwide, the temporal drift between the calendar and the seasons is mitigated, ensuring continued accuracy in timekeeping.
Leap years are essential adjustments in the calendar systems to account for the fractional excess in the Earth's orbit around the sun. As the solar year is longer than the typical 365-day calendar year, the addition of an extra day on February 29th during leap years in the Gregorian calendar compensates for this discrepancy, thereby maintaining temporal consistency.
It is worth noting that leap years are not exclusive to the Gregorian calendar but are also incorporated in other systems like the Julian calendar, highlighting their universal importance in accurately measuring time.
Conclusion
This Python program checks if a given year is a leap year or not. It prompts the user to input a year and then determines if it is divisible by 4 but not divisible by 100, or if it is divisible by 400, making it a leap year if either condition is met. The program then outputs whether the year is a leap year or not.
- Print the following pattern using python
- Remove first n characters from a string | Python
- Check if the first and last number of a list is the same | Python
- Number of occurrences of a substring in a string in Python
- Remove Last Element from List in Python
- How to Use Modulo Operator in Python
- Enumerate() in Python
- Writing to a File with Python's print() Function
- How to read csv file in Python
- Dictionary Comprehension in Python
- How to Convert List to String in Python
- How to convert int to string in Python
- Random Float numbers in Python