Check if String Contains Substring in Python
Verifying the presence of a substring within a string is a frequent and fundamental task in various programming languages. Python provides a diverse array of methods to tackle this task effectively. The most straightforward and efficient approach in Python involves utilizing the "in" operator as a comparison operator, enabling quick determination of substring existence.
Additionally, Python offers other useful methods like find(), index(), and count() to further facilitate substring checking and analysis. By employing these methods wisely, programmers can efficiently and accurately ascertain the presence and characteristics of substrings within a given string.
Using Python's "in" operator
The most straightforward and efficient approach to check whether a string contains a substring in Python is to use the "in" operator. This operator evaluates to true if the substring is present in the string, otherwise, it returns false.
The "in" operator in Python takes two arguments: one on the left and one on the right. It evaluates to True if the left argument, which represents a substring, is contained within the right argument, which represents the original string. If the substring is found, the "in" operator returns True; otherwise, it returns False.
More on "in" operator
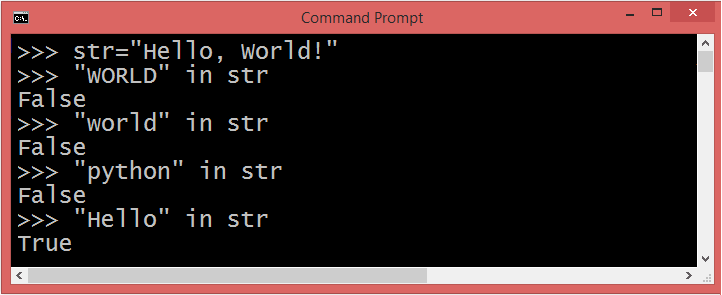
Note: The "in" operator is case sensitive i.e, it will treat the Uppercase characters and Lowercase characters differently.
__contains__() function
In Python, the String class has the __contains__() method, which allows us to check if one string contains another. When we use the "in" operator with strings, it internally calls the __contains__() method to determine the containment.
Although we can directly use the __contains__() method, it is considered semantically private (also known as "dunder" methods) and should not be used directly in regular code. Instead, it is recommended to use the "in" operator for readability and better code maintainability.
Using Python's str.find() method
Another method available for checking substring presence in Python is the find() method of the string class. This method evaluates whether the substring is contained within the string. If the substring is found, the find() method returns the starting index of the first occurrence of the substring within the string; otherwise, it returns -1, signifying that the substring is not present. This feature proves beneficial when you need to determine the specific position of the substring within the string for further analysis or manipulation.
More on find() method
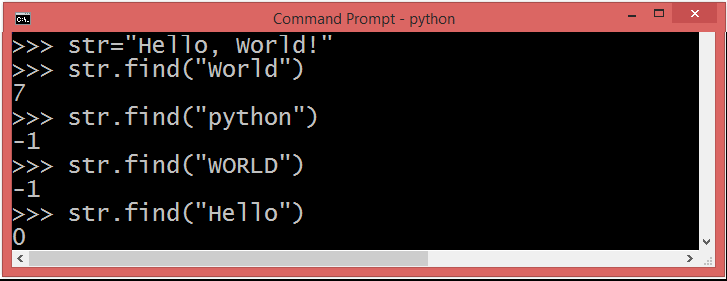
While using the str.find() method to check for substring presence is considered less Pythonic compared to using the "in" operator or other more concise methods, it is still an accepted approach. The str.find() method is slightly longer and can be more confusing for some, but it remains a valid and functional way to achieve the desired result. Despite its drawbacks, it still serves the purpose of determining the starting index of the first occurrence of the substring within the string or returning -1 if the substring is not present. Although it may not be the most idiomatic approach in Python, it remains a viable option for certain scenarios.
Using Python Regular Expression
Regular expressions (regex) are widely used for pattern matching in various programming tasks, including text parsing, data extraction, and validation. Python provides a built-in module called re, which offers powerful capabilities to work with regular expressions.
The re module in Python includes a function called search(), which allows you to check if a string contains a specified search pattern using regular expressions. The search() function scans the input string and looks for the first occurrence of the specified pattern. If the pattern is found, it returns a match object; otherwise, it returns None.
Using str.count() method
If you wish to determine the frequency of a particular substring occurrence within a string, Python provides the count() method for this purpose. Utilizing the count() method allows you to obtain an accurate count of the occurrences of the specified substring. In cases where the substring is not present in the string, the function returns 0, signifying the absence of the substring. Employing the count() method effectively aids in analyzing the distribution and repetition of substrings within the given text data.
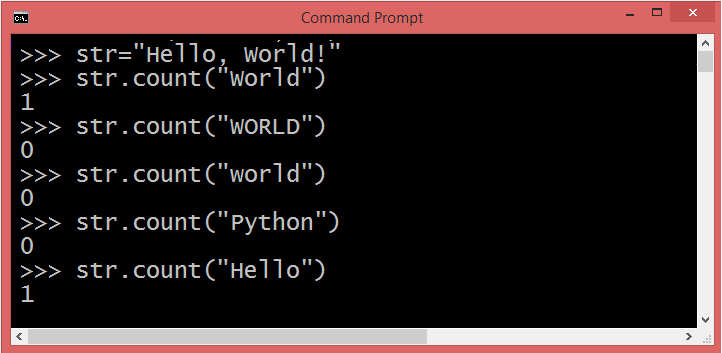
Conclusion
Each of these methods has its own advantages and use cases. The "in" operator is the simplest and most readable for basic checks, while str.find() allows you to get the index of the first occurrence of the substring. The re.search() function is useful for more advanced pattern matching using regular expressions, and str.count() provides a count of occurrences of the substring. Choose the appropriate method based on your specific needs for checking if a string contains a substring in Python.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples