f-strings | String Formatting in Python
F-strings, also known as "formatted string literals," are a feature introduced in Python 3.6 that allows you to embed expressions inside string literals. They provide a concise and readable way to create strings that include the values of variables or the results of expressions without the need for concatenation or string formatting functions.
To create an f-string, you simply prefix the string literal with the letter 'f' or 'F'. Inside the f-string, you can include expressions inside curly braces {}. The expressions inside the curly braces are evaluated at runtime and their values are substituted into the final string.
Expressions in F-strings
F-strings can include any valid Python expression inside the curly braces. This allows you to perform calculations, access attributes of objects, and call functions directly within the f-string.
Formatting Options
F-strings can also include formatting options to control the display of numeric values, such as specifying the number of decimal places or aligning the values.
Escaping Curly Braces
If you need to include literal curly braces in the f-string, you can escape them by doubling them ({{ and }}).
f-strings Vs format()
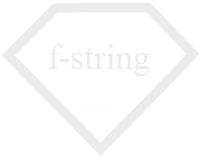
There's a bunch of ways to handle string formatting in Python. f-string strings were introduced to address some of the shortcomings other methods for formatting strings.
3 key reasons why f-Strings are better.
- #1 — f-Strings look clean.
- #2 — f-Strings are faster.
- #3 — f-Strings allow extensive manipulation.
F-Strings, available in Python 3.6 and later versions, are the preferred choice for string formatting due to their concise and efficient nature. If you have the opportunity to work with Python 3.6 or newer, it is recommended to use f-Strings for improved readability and maintainability. However, for scenarios where compatibility with earlier versions is necessary, the format() method remains a viable alternative, ensuring seamless execution across different Python versions while still achieving dynamic string formatting.
Conclusion
F-strings offer a concise and powerful way to create dynamic strings in Python, making code more readable and maintainable. However, please note that f-strings are available in Python 3.6 and above, so if you are using an earlier version, you'll need to use other string formatting methods like str.format() or %.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- What is a None value in Python?
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples