What is the None keyword in Python?
In Python, None is a special keyword that represents the absence of a value. It is used to signify that a variable or object does not have a value assigned to it. In other words, it is a way to represent "nothing" or "null" in Python.
Use of None
- When you create a variable without assigning any value, it is automatically initialized to None.
- Functions that do not explicitly return a value return None by default.
- It can be used as a placeholder when you want to define a variable or object initially without assigning a specific value.
Comparison with None
You can use the is or is not operators to compare variables with None to check if they are holding the None value.
ExampleReturning None from Functions
If a function has no explicit return statement or returns without a value, it automatically returns None. It is common to use None as a return value to indicate that a function has no meaningful result to return.
ExampleIn this example, the divide function returns None when the divisor is 0, indicating that division by zero is not allowed.
Python NoneType
None is a unique object and the sole instance of the class NoneType. Attempts to create additional instances of NoneType will always return the same None object, making it a singleton. This distinct characteristic of None ensures that there is only one instance of this object throughout the program's execution. As a favored baseline value, None is frequently employed to represent the absence of a meaningful value in various algorithms and scenarios within the language and library. Its usage as an exceptional value allows for concise and expressive code, providing a clear indication of missing or unspecified information.
Checking if a variable is None using is operator:
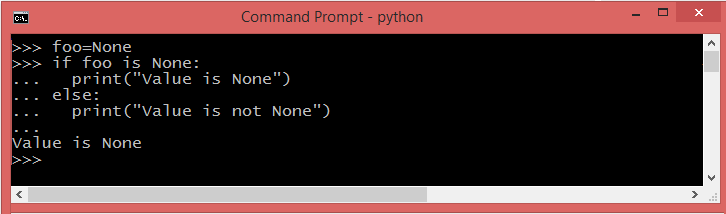
Assigning a value of None to a variable is one way to reset it to its original, empty state. It is not the same as 0, False, or an empty string. None is a data type just like int, float, etc. and only None can be None. You can check out the list of default types available in Python in 8.15. types — Names for built-in types.
So, let's check how Python None implemented internally.
- Except __setattr__, all others are read-only attributes. So, there is no way we can alter the attributes of None.
Checking if a variable is None using == operator:
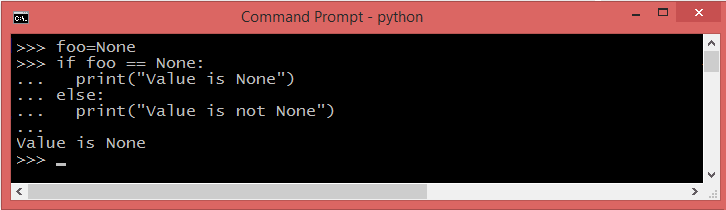
Python Null and None Keyword
There is no "null" keyword like in some other programming languages. Instead, Python uses the None object to represent the absence of a value. The None object is a singleton, meaning there is only one instance of it throughout the program's execution. You can verify its uniqueness by using Python's identity function id(), which returns a unique number assigned to each object. If the id of two variables is the same, it confirms that they both point to the same None object.
Comparing None with False type:
Interesting Facts about Python None
- None is an instance of NoneType.
- We cannot create other instances of NoneType.
- None is not the same as False.
- None cannot have new attributes.
- Existing attributes of None cannot be changed.
- None is not an empty string.
- None is not 0.
- If the function doesn't return anything that means its None in Python.
- We cannot even change the reference to None by assigning values to it.
- Comparing None to anything will always return False except None itself.
Conclusion
The use of None instead of "null" is a design choice in Python, and it serves as a clear indication of missing or undefined values. By using the identity function id() to check object uniqueness, developers can ensure that variables indeed refer to the same None object, providing a reliable way to handle absence of values in Python programs.
- Keywords in Python
- Python Operator - Types of Operators in Python
- Python Data Types
- Python Shallow and deep copy operations
- Python Datatype conversion
- Python Mathematical Function
- Basic String Operations in Python
- Python Substring examples
- How to check if Python string contains another string
- Check if multiple strings exist in another string : Python
- Memory Management in Python
- Python Identity Operators
- How to Install a Package in Python using PIP
- How to update/upgrade a package using pip?
- How to Uninstall a Package in Python using PIP
- How to call a system command from Python
- How to use f-string in Python
- Python Decorators (With Simple Examples)
- Python Timestamp Examples