UnboundLocalError: local variable referenced before assignment
The unboundlocalerror: local variable referenced before assignment is raised when you try to use a variable before it has been assigned in the local context. Python doesn't have variable declarations , so it has to figure out the scope of variables itself. It does so by a simple rule: If there is an assignment to a variable inside a function, that variable is considered local .
For ex:
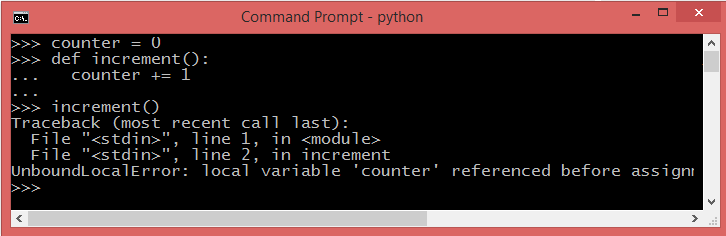
If you set the value of a variable inside the function , python understands it as creating a local variable with that name. This local variable masks the global variable .
Here, the line
implicitly makes "counter" local to increment(). Trying to execute this line, though, will try to read the value of the local variable "counter" before it is assigned, resulting in an UnboundLocalError . To solve this problem, you can explicitly say it's a global by putting global declaration in you function.
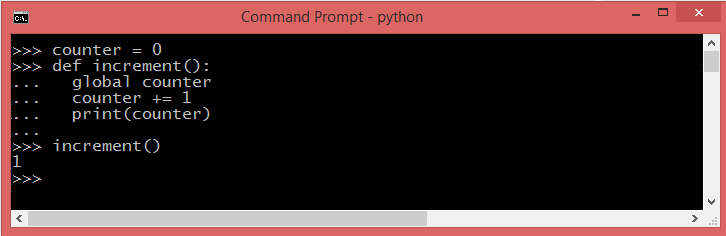
The global statement does not have to be at the beginning of the function definition, but that is where it is usually placed. Wherever it is placed, the global declaration makes a variable to global variable everywhere in the function. If increment() is a local function and counter a local variable, you can use "nonlocal" in Python 3.x. This keyword is useful when we need to assign any value to nested scope variable.
Python has lexical scoping by default, which means that although an enclosed scope can access values in its enclosing scope, it cannot modify them (unless they're declared global with the global keyword). All variable assignments in a function store the value in the local symbol table; whereas variable references first look in the local symbol table, then in the global symbol table, and then in the table of built-in names. Thus, global variables cannot be directly assigned a value within a function (unless named in a global statement), although they may be referenced.
- TypeError: 'NoneType' object is not subscriptable
- IndexError: string index out of range
- IndentationError: unexpected indent Error
- ValueError: too many values to unpack (expected 2)
- SyntaxError- EOL while scanning string literal
- TypeError: Can't convert 'int' object to str implicitly
- IndentationError: expected an indented block
- ValueError: invalid literal for int() with base 10
- IndexError: list index out of range : Python
- AttributeError: 'module' object has no attribute 'main'
- TypeError: string indices must be integers
- FileNotFoundError: [Errno 2] No such file or directory
- Fatal error: Python.h: No such file or directory
- ZeroDivisionError: division by zero
- ImportError: No module named requests | Python
- TypeError: 'NoneType' object is not iterable
- SyntaxError: unexpected EOF while parsing | Python
- zsh: command not found: python
- Unicodeescape codec can't decode bytes in position 2-3
- The TypeError: 'tuple' object does not support item assignment
- The AttributeError: 'bytes' object has no attribute 'read'